Meddelanden på plats
Meddelanden på plats tillhandahåller ett omedelbart sätt för dig att underrätta användare om någonting som hände medan programmet användes.
Grundläggande meddelande på plats
Kirigami.InlineMessage komponenter har två viktiga egenskaper att vara medveten om:
- visible: Normalt är den inställd till
false
, så att meddelandet bara visas när du explicit vill det. Det kan överskridas om du vill genom att ställa in den tilltrue
. När ett dolt meddelande på plats ställs in att vara synligt, får du en snygg animering. - text: Här är platsen där du anger texten för meddelandet på plats
import QtQuick
import QtQuick.Layouts
import QtQuick.Controls as Controls
import org.kde.kirigami as Kirigami
Kirigami.Page {
ColumnLayout {
Kirigami.InlineMessage {
id: inlineMessage
Layout.fillWidth: true
text: "Hello! I am a siiiiiiimple lil' inline message!"
}
Controls.Button {
text: "Show inline message!"
onClicked: inlineMessage.visible = !inlineMessage.visible
}
}
}
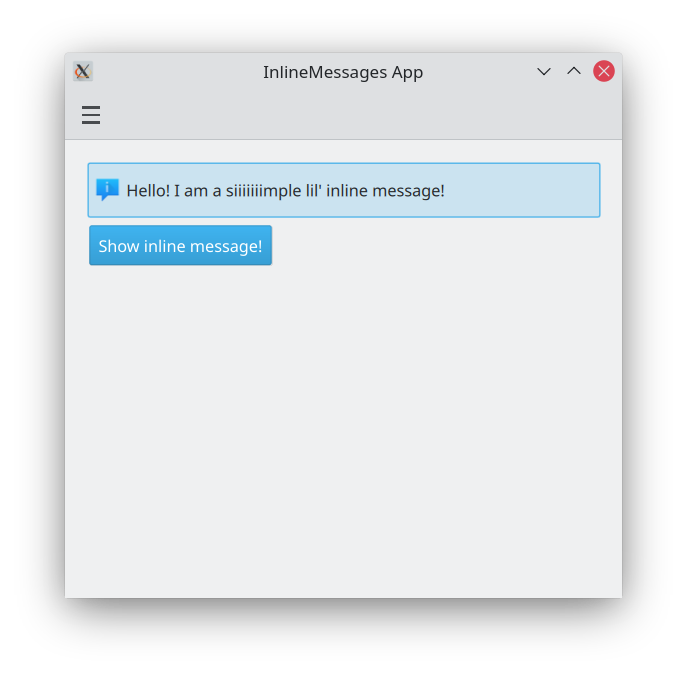
Olika typer
Vanliga meddelande på plats är som de ovan: de har en blå bakgrund och en standardikon. Vi kan ändra det med egenskapen type, som låter oss ställa in våra meddelande på plats till en annan typ. Det finns fyra typer vi kan välja mellan:
- Information (
Kirigami.MessageType.Information
): förval. Har en blå bakgrund, en 'i' ikon, och används för att kungöra ett resultat eller tala om något generellt för användaren. Det är inte nödvändigt att ställa in det manuellt. - Positiv (
Kirigami.MessageType.Positive
): har en grön bakgrund, bockikon, och anger att något gick bra. - Varning (
Kirigami.MessageType.Warning
): har en orange background, en utropsteckenikon, och kan användas för att varna användaren för någonting de bör vara medvetna om. - Fel (
Kirigami.MessageType.Error
): har en röd bakgrund, en korsikon, och kan användas för att tala om för användaren att någonting har gått fel.
ColumnLayout {
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hello! Let me tell you something interesting!"
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hey! Let me tell you something positive!"
type: Kirigami.MessageType.Positive
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hmm... You should keep this in mind!"
type: Kirigami.MessageType.Warning
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Argh!!! Something went wrong!!"
type: Kirigami.MessageType.Error
visible: true
}
}
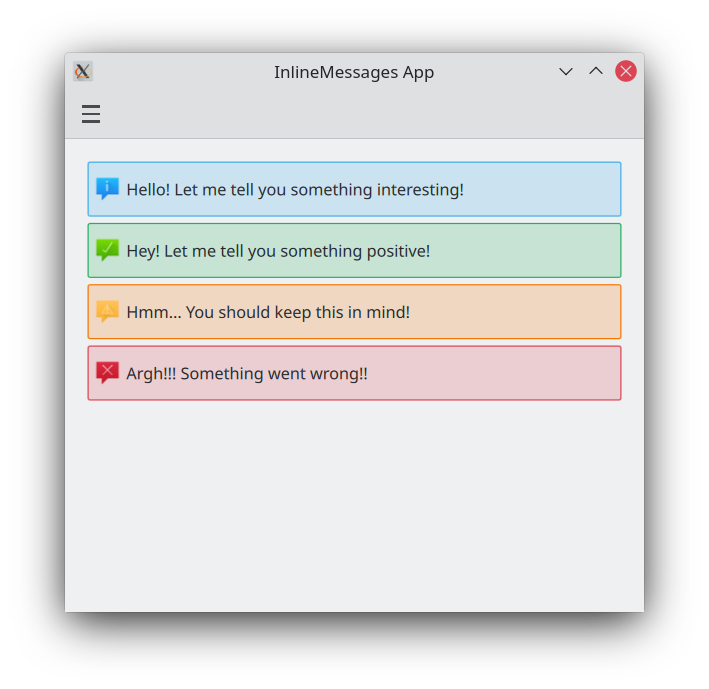
Anpassa text och ikoner
Meddelande på plats stöder rich text, som kan definieras med enkel HTML-liknande taggar. Det låter oss lägga till viss formatering i dina meddelandetexter eller till och med inkludera en extern webblänk om du vill.
Kirigami.InlineMessage {
Layout.fillWidth: true
// Observera att när du använder citationstecken i en sträng måste de undantas.
text: "Check out <a href=\"https://kde.org\">KDE's website!<a/>"
onLinkActivated: Qt.openUrlExternally(link)
visible: true
}
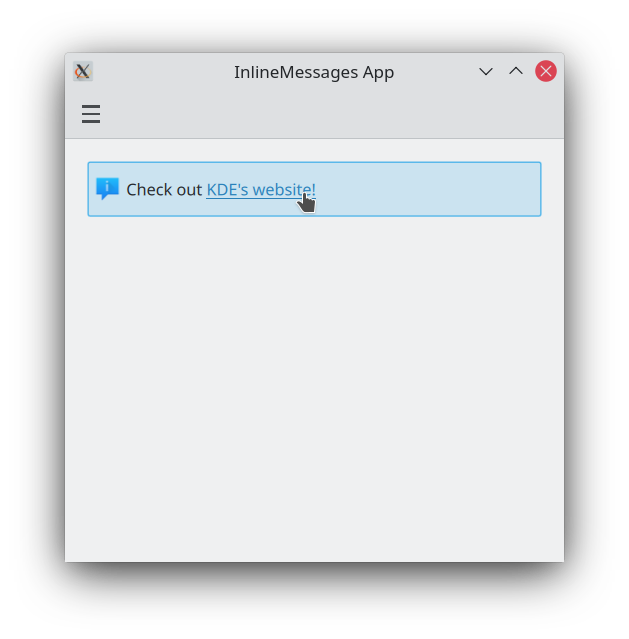
Du kan också anpassa ikonen som visas längst upp till vänster i meddelandet genom att tillhandahålla ett systemikonnamn för egenskapen icon.source. Ikonnamnen ska motsvara ikoner installerade på systemet. Du kan använda ett program såsom Cuttlefish som tillhandahålls av plasma-sdk för att bläddra och söka efter tillgängliga ikoner på systemet, och vad deras namn är.
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Look at me! I look SPECIAL!"
icon.source: "actor"
visible: true
}
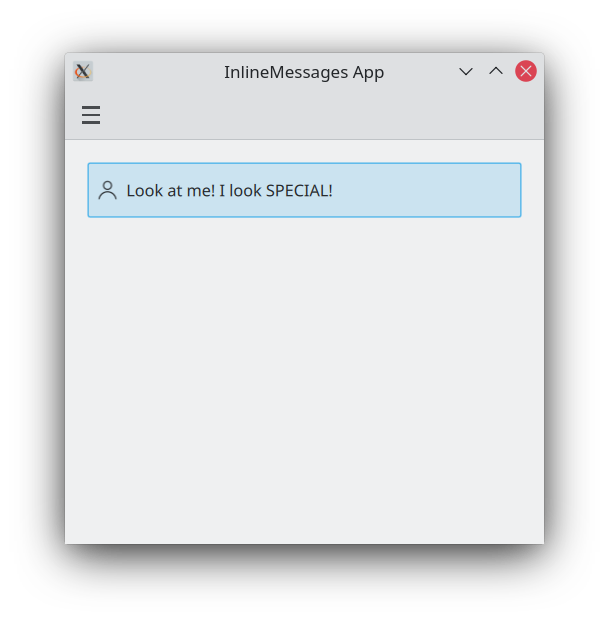
Använda åtgärder i meddelanden på plats
Om dina meddelanden måste vara interaktiva, kan du koppla Kirigami-åtgärder till dina meddelanden på plats. Liksom med sidor, kan du göra det genom att ställa in meddelandets egenskap InlineMessage.actions antingen en Kirigami.Action eller ett fält som innehåller Kirigami.Action komponenter.
ColumnLayout {
Kirigami.InlineMessage {
id: actionsMessage
Layout.fillWidth: true
visible: true
readonly property string initialText: "Something is hiding around here..."
text: initialText
actions: [
Kirigami.Action {
enabled: actionsMessage.text == actionsMessage.initialText
text: qsTr("Add text")
icon.name: "list-add"
onTriggered: {
actionsMessage.text = actionsMessage.initialText + " Peekaboo!";
}
},
Kirigami.Action {
enabled: actionsMessage.text != actionsMessage.initialText
text: qsTr("Reset text")
icon.name: "list-remove"
onTriggered: actionsMessage.text = actionsMessage.initialText
}
]
}
}
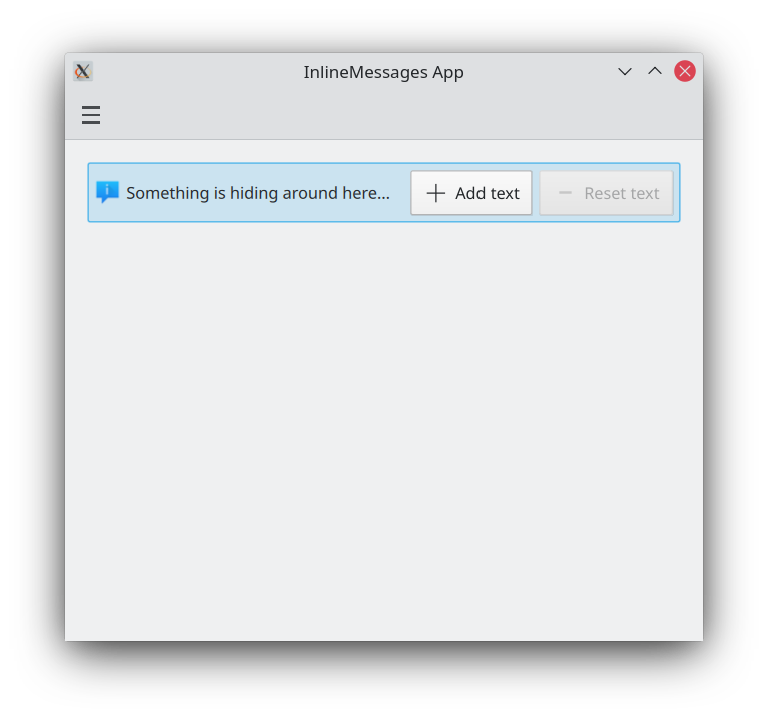
Stängningsknappar
Meddelanden på plats tillhandahåller en stängningsknapp som kan användas för att enkelt avfärda dem.
Normalt är stängningsknappen dold, men det kan överskridas genom att ställa in egenskapen showCloseButton till true
.
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Please don't dismiss me..."
showCloseButton: true
visible: true
}
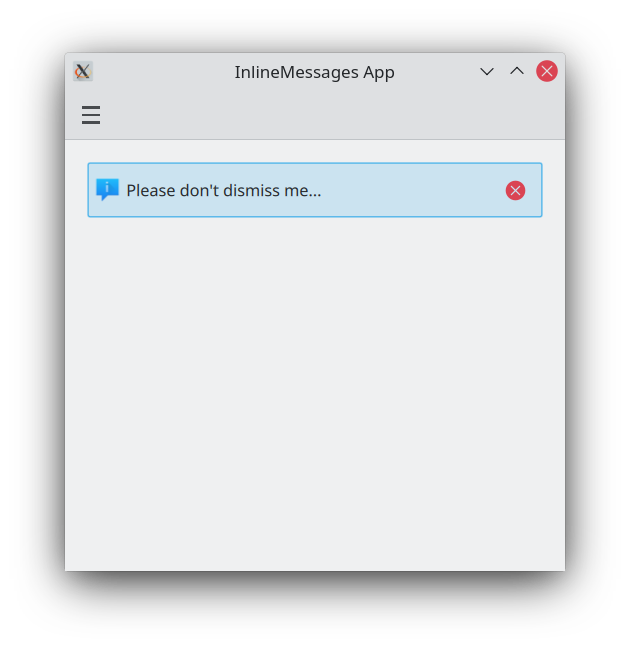