Mensajes en línea
Los mensajes en línea proporcionan un modo inmediato para que pueda notificar a los usuarios sobre algo que haya ocurrido durante el uso de la aplicación.
Mensaje en línea básico
Los componentes Kirigami.InlineMessage tienen dos propiedades importantes a tener en cuenta:
- visible: tiene el valor
false
de forma predeterminada, por lo que el mensaje solo aparece cuando se indique de forma explícita. Este comportamiento se puede modificar asignándole el valortrue
. Cuando un mensaje en línea oculto se define como visible, se mostrará con una bonita animación. - text : aquí es donde se define el texto del mensaje en línea.
import QtQuick
import QtQuick.Layouts
import QtQuick.Controls as Controls
import org.kde.kirigami as Kirigami
Kirigami.Page {
ColumnLayout {
Kirigami.InlineMessage {
id: inlineMessage
Layout.fillWidth: true
text: "Hello! I am a siiiiiiimple lil' inline message!"
}
Controls.Button {
text: "Show inline message!"
onClicked: inlineMessage.visible = !inlineMessage.visible
}
}
}
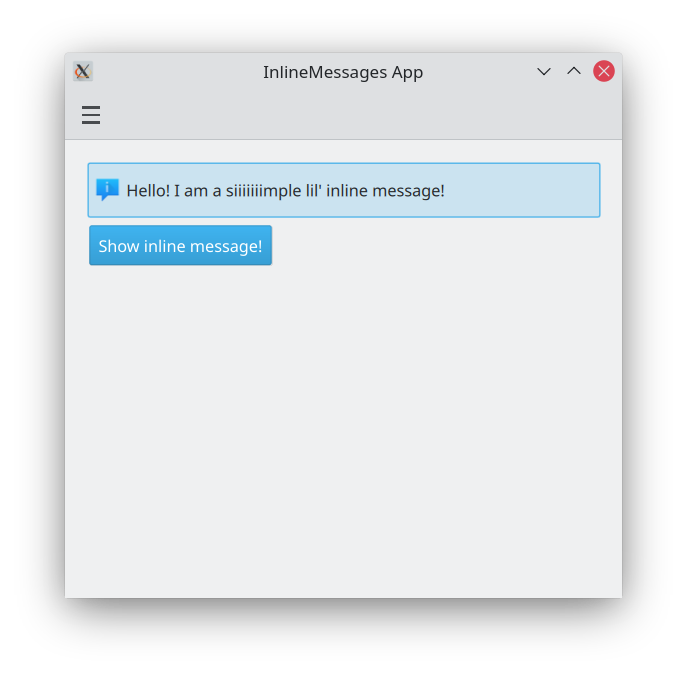
Diferentes tipos
Los mensajes en línea estándares son como los anteriores: tienen un fondo azul y un icono predeterminado. Podemos cambiar esto con la propiedad type , que nos permite hacer que nuestro mensaje en línea sea de un tipo diferente. Hay cuatro tipos entre los que podemos elegir:
- Información (
Kirigami.MessageType.Information
): el predeterminado. Tiene un fondo azul, un icono 'i' y se usa para anunciar un resultado o para comunicarle al usuario algo general. No es necesario definirlo manualmente. - Positivo (
Kirigami.MessageType.Positive
): tiene un fondo verde, un icono con una marca de verificación e indica que algo ha ido bien. - Advertencia (
Kirigami.MessageType.Warning
): tiene un fondo naranja, un icono con un signo de exclamación y se puede usar para advertir al usuario sobre algo que debería tener en cuenta. - Error (
Kirigami.MessageType.Error
): tiene un fondo rojo, un icono con una equis y se puede usar para comunicar al usuario que algo ha ido mal.
ColumnLayout {
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hello! Let me tell you something interesting!"
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hey! Let me tell you something positive!"
type: Kirigami.MessageType.Positive
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Hmm... You should keep this in mind!"
type: Kirigami.MessageType.Warning
visible: true
}
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Argh!!! Something went wrong!!"
type: Kirigami.MessageType.Error
visible: true
}
}
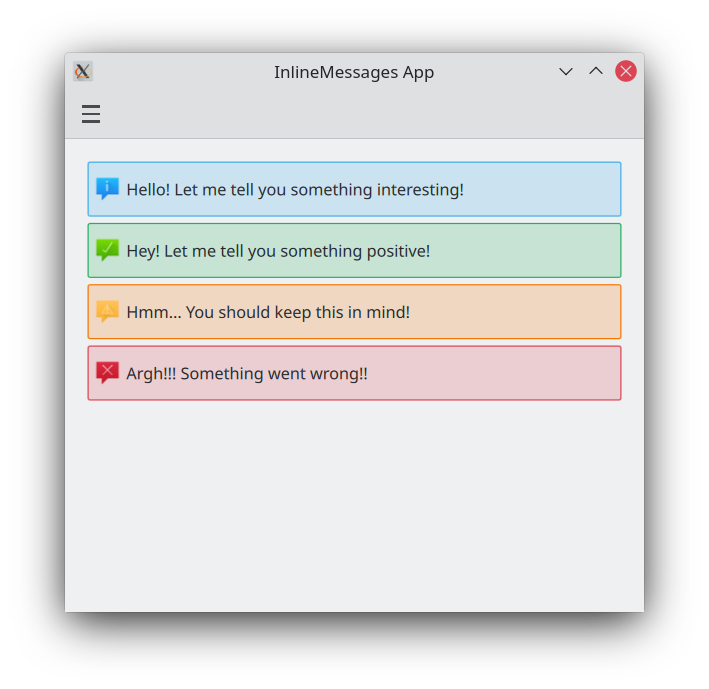
Personalización de texto y de iconos
Los mensajes en línea permiten el uso de texto enriquecido, que puede constar de etiquetas sencillas de tipo HTML. Esto le permite añadir cierto formato al texto de los mensajes en línea e incluso insertar enlaces web externos, si así lo desea.
Kirigami.InlineMessage {
Layout.fillWidth: true
// Tenga en cuenta que cuando use comillas en una cadena de texto, tendrá que usar secuencias de
// escape con ellas.
text: "Check out <a href=\"https://kde.org\">KDE's website!<a/>"
onLinkActivated: Qt.openUrlExternally(link)
visible: true
}
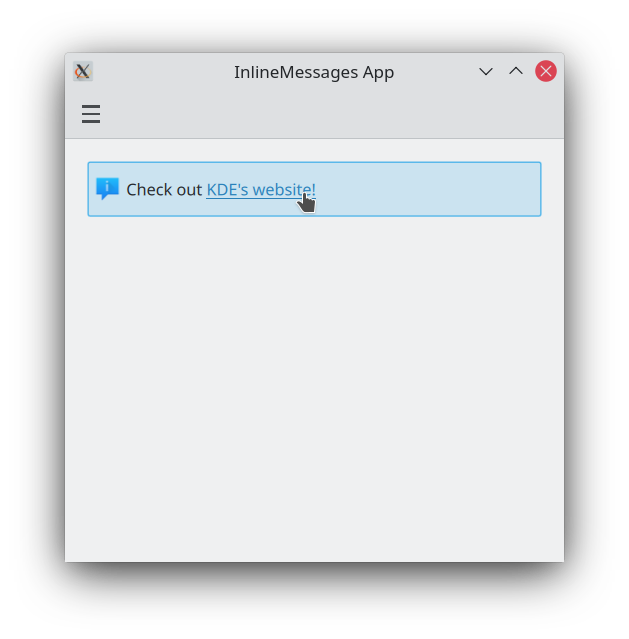
You can also customise the icon that appears on the top left of your message by providing a system icon name for the icon.source property. These icon names should correspond to icons installed on your system; you can use an application such as Cuttlefish provided by plasma-sdk to browse and search the icons available on your system, and see what their names are.
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Look at me! I look SPECIAL!"
icon.source: "actor"
visible: true
}
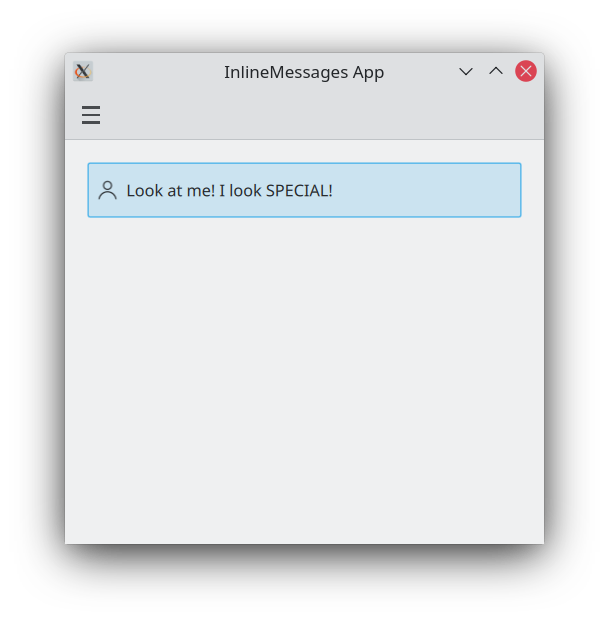
Uso de acciones en mensajes en línea
If your messages need to be interactive, you can attach Kirigami actions to your inline messages. Like with pages, you can do this by setting the InlineMessage.actions property to either a Kirigami.Action or an array containing Kirigami.Action components.
ColumnLayout {
Kirigami.InlineMessage {
id: actionsMessage
Layout.fillWidth: true
visible: true
readonly property string initialText: "Something is hiding around here..."
text: initialText
actions: [
Kirigami.Action {
enabled: actionsMessage.text == actionsMessage.initialText
text: qsTr("Add text")
icon.name: "list-add"
onTriggered: {
actionsMessage.text = actionsMessage.initialText + " Peekaboo!";
}
},
Kirigami.Action {
enabled: actionsMessage.text != actionsMessage.initialText
text: qsTr("Reset text")
icon.name: "list-remove"
onTriggered: actionsMessage.text = actionsMessage.initialText
}
]
}
}
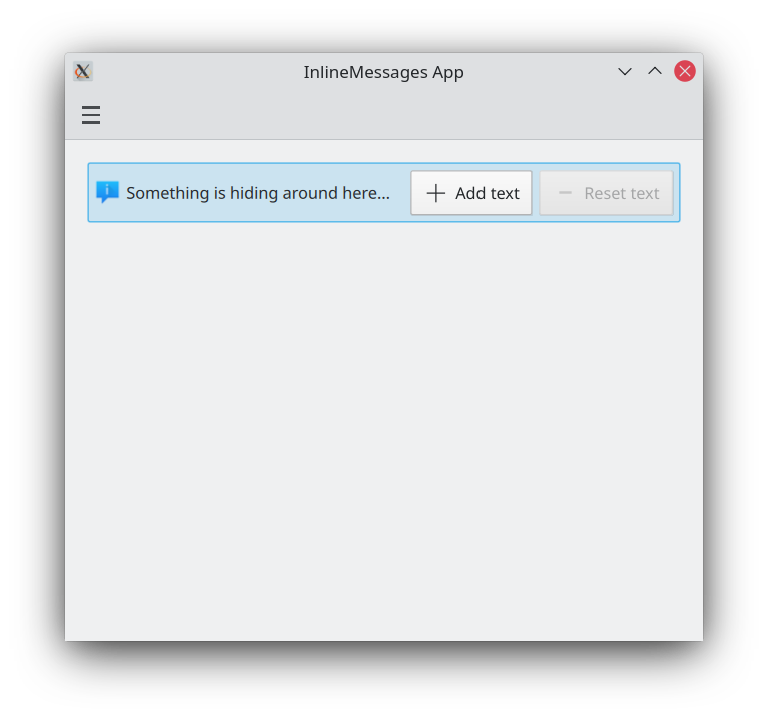
Botones de cierre
Los mensajes en línea proporcionan un botón de cierre que se puede usar para descartarlos fácilmente.
De forma predeterminada, este botón de cierre está oculto, pero puede modificar este comportamiento definiendo la propiedad
showCloseButton
a true
.
Kirigami.InlineMessage {
Layout.fillWidth: true
text: "Please don't dismiss me..."
showCloseButton: true
visible: true
}
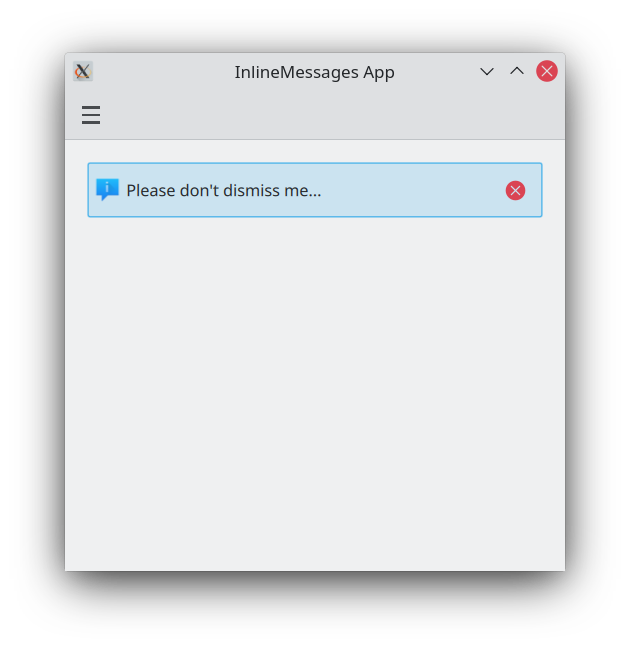