Diseños de formulario
Kirigami.FormLayout components make it easy for you to create forms that conform to the KDE Human Interface Guidelines. They are optimal for settings dialogs and for large groups of controls and input fields that are related to each other.
Cuando se les proporciona suficiente espacio, los diseños de formularios ocuparán dos columnas. La columna de la izquierda estará ocupada con las etiquetas proporcionadas para los componentes secundarios del formulario, mientras que la derecha contendrá los propios elementos secundarios. En ventanas con espacio más limitado (o en dispositivos móviles), los formularios constarán de una sola columna vertical con las etiquetas de los componentes secundarios colocadas sobre sus respectivos componentes.
Formulario sencillo
Kirigami.FormLayout components are similar in use to QtQuick Layout components such as ColumnLayout or RowLayout. The child components will be automatically arranged according to the size available to the form layout.
Children of a Kirigami.FormLayout have a property named Kirigami.FormData.label. This property lets you set the label that will be provided for the child component in question.
import QtQuick
import QtQuick.Layouts
import QtQuick.Controls as Controls
import org.kde.kirigami as Kirigami
Kirigami.ApplicationWindow {
pageStack.initialPage: Kirigami.Page {
Kirigami.FormLayout {
anchors.fill: parent
Controls.TextField {
Kirigami.FormData.label: "TextField 1:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 2:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 3:"
}
}
}
}
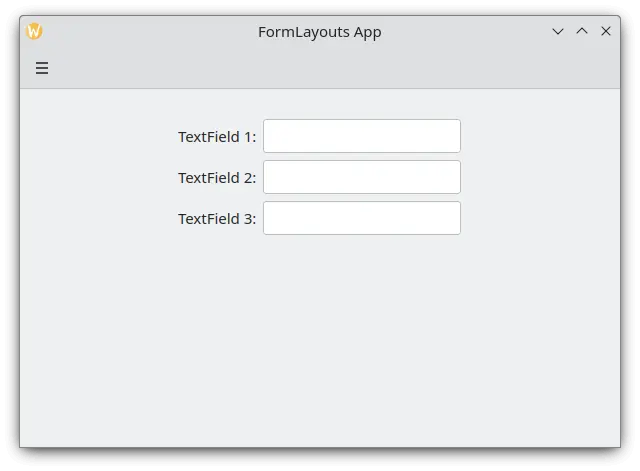
Un diseño de formulario simple en modo escritorio
Secciones y separadores
FormLayouts can also be divided into sections. Setting where a section starts is as easy as setting a child component's Kirigami.FormData.isSection to true. This will provide the component with some extra margin at the top to demarcate the start of the new section.
Kirigami.Separator components are best suited for starting new sections. Separators are used to draw a thin horizontal line, demarcating the end of a section. If you would rather not have a line drawn between sections, you can use a standard QML Item property. Alternatively you could use the Kirigami.FormData.isSection property on any other component.
However, this is not recommended. On components where Kirigami.FormData.isSection is set to true
, the label text provided for this component's Kirigami.FormData.label property will be displayed as the section's header text.
Advertencia
This does not apply to every component, hence the recommendation that you use Kirigami.Separator or Item components in places where you would like to use a header text.Este texto de encabezado es más grande que el texto de etiqueta normal y proporciona al usuario una buena pista visual sobre de qué trata la sección de diseño del formulario.
Kirigami.FormLayout {
anchors.fill: parent
Controls.TextField {
Kirigami.FormData.label: "TextField 1:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 2:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 3:"
}
Kirigami.Separator {
Kirigami.FormData.isSection: true
Kirigami.FormData.label: "New Section!"
}
ColumnLayout {
Kirigami.FormData.label: "Radio buttons"
Controls.RadioButton {
text: "Radio 1"
checked: true
}
Controls.RadioButton {
text: "Radio 2"
}
Controls.RadioButton {
text: "Radio 3"
}
}
Item {
Kirigami.FormData.isSection: true
Kirigami.FormData.label: "Another Section! (lineless though)"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 4:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 5:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 5:"
}
}
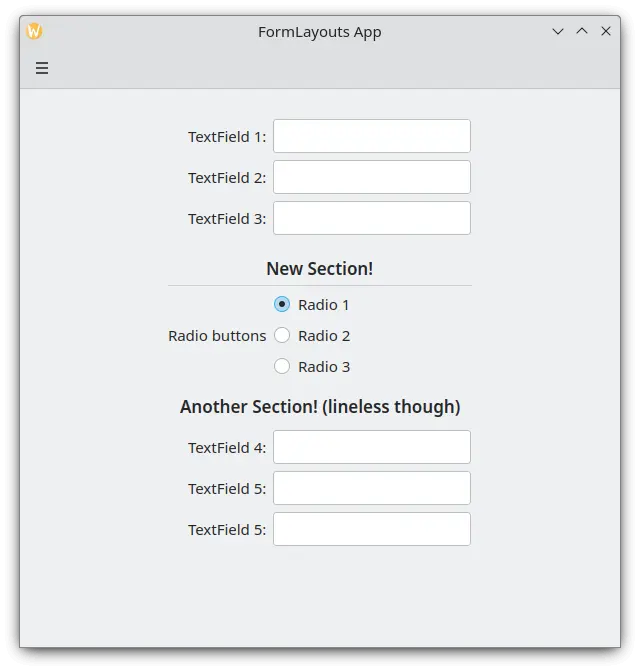
Un diseño de formulario con secciones
Hijos con casillas de verificación
A handy feature of Kirigami.FormLayout is that you can add checkboxes to its children. This can be useful in settings pages where you might want to let the user enable or disable a setting, and also want the user to provide some extra information in a component such as a textfield.
Kirigami.FormLayout {
anchors.fill: parent
Controls.TextField {
Kirigami.FormData.label: "First name:"
}
Controls.TextField {
Kirigami.FormData.label: "Middle name:"
Kirigami.FormData.checkable: true
enabled: Kirigami.FormData.checked
}
Controls.TextField {
Kirigami.FormData.label: "Last name:"
}
}
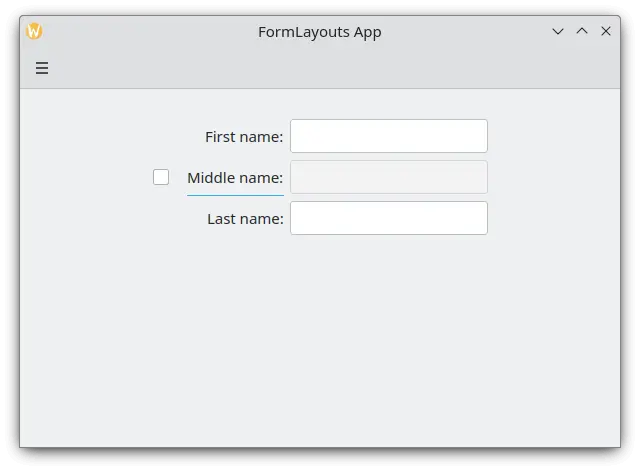
Un diseño de formulario con una etiqueta que se puede marcar.
Forzar un diseño de escritorio o móvil
If you would rather have your form layout stay consistent regardless of your application's environment, you can use the wideMode property of the Kirigami.FormLayout component:
- Cuando vale
true
, el formulario se estructurará en formato de pantalla ancha optimizado para el escritorio (a doble columna) - Cuando vale
false
, el formulario se organizará en un formato para móviles (a una sola columna)
Kirigami.FormLayout {
anchors.fill: parent
wideMode: false
Controls.TextField {
Kirigami.FormData.label: "TextField 1:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 2:"
}
Controls.TextField {
Kirigami.FormData.label: "TextField 3:"
}
}
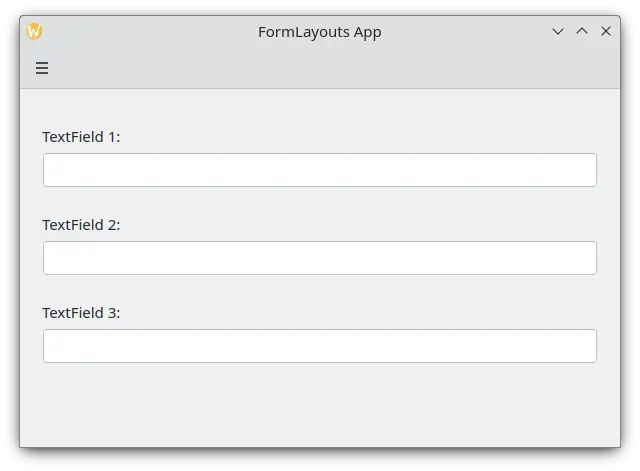
Un diseño de formulario con diseño móvil forzado
Alineación de etiquetas
There are instances when you want a label to be assigned to components that have more than one line or to a list of components. This can be achieved by putting the Kirigami.FormData.label in the ColumnLayout, as you might have noticed in Sections and Separators. By default the label is positioned in the vertical center of the layout, which is not always desirable. We can change this with help of Kirigami.FormData.labelAlignment.
Kirigami.FormLayout {
anchors.fill: parent
ColumnLayout {
Kirigami.FormData.label: "This is a label:"
Kirigami.FormData.labelAlignment: Qt.AlignTop
Controls.Label {
text: "This is some rather long text \nthat should elide to multiple lines \nto show how the label gets aligned."
elide: Text.elideLeft
}
}
}
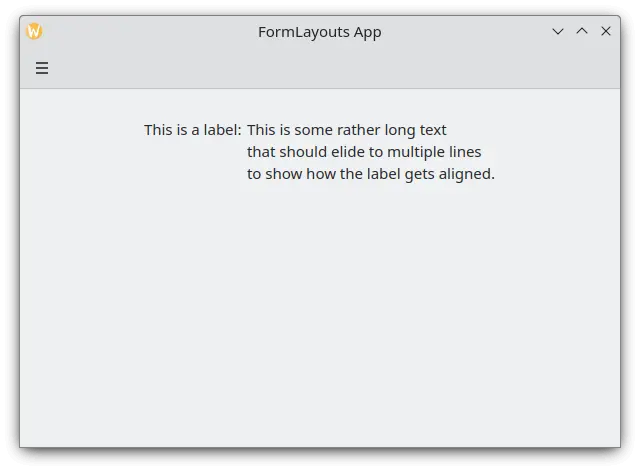
Un diseño de formulario con una etiqueta alineada a la parte superior
Setting the label alignment is particularly convenient to manage components or lists of components whose size you do not know beforehand. Elisa is a very good example of this:
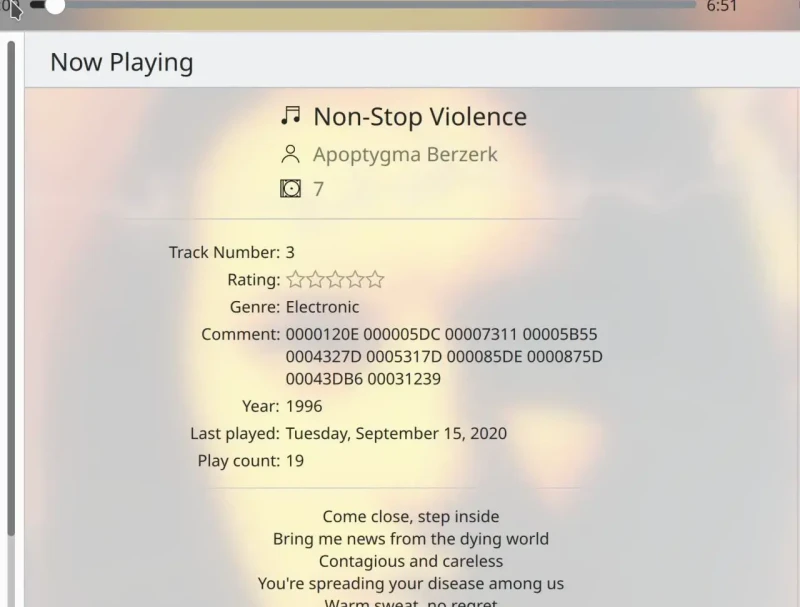
La etiqueta Comment solo está alineada a la parte superior cuando su correspondiente componente tiene más de una línea.
Podemos hacer algo similar a esto con un operador ternario de JavaScript:
Kirigami.FormLayout {
anchors.fill: parent
ColumnLayout {
Kirigami.FormData.label: "This is a label:"
Kirigami.FormData.labelAlignment: labelText.text.lineCount > 1 ? Qt.AlignTop : Qt.AlignVCenter
Controls.Label {
id: labelText
text: "This is some rather long text \nthat should elide to a new line \nso it appears below the Form Label."
elide: Text.elideLeft
}
}
}