Componentes basados en acciones
Acciones
Una Kirigami.Action consiste en una acción sobre la que se puede hacer clic y cuya apariencia depende de donde se añada. Por lo general es un botón con un icono y un texto.
Podemos usarlas para proporcionar a nuestras aplicaciones acciones de fácil acceso que son esenciales para su funcionalidad.
Nota
Las acciones de Kirigami heredan de QtQuick.Controls.Action y se les pueden asignar atajos de teclado.Al igual que las acciones de los controles de QtQuick , se pueden asignar a elementos del menú y a botones de la barra de herramientas, así como a otros componentes de Kirigami.
import org.kde.kirigami as Kirigami
Kirigami.Action {
id: copyAction
text: i18n("Copy")
icon.name: "edit-copy"
shortcut: StandardKey.Copy
onTriggered: {
// ...
}
}
Nota
La propiedad icon.name usa nombres de iconos del sistema siguiendo la especificación de FreeDesktop. Estos iconos y nombres de iconos se pueden ver con la aplicación CuttleFish de KDE, incluida en plasma-sdk, o visitando la especificación de nombres de iconos de FreeDesktop.One feature offered by Kirigami Actions on top of QtQuick Actions is the possibility to nest actions.
import org.kde.kirigami as Kirigami
Kirigami.Action {
text: "View"
icon.name: "view-list-icons"
Kirigami.Action {
text: "action 1"
}
Kirigami.Action {
text: "action 2"
}
Kirigami.Action {
text: "action 3"
}
}
Otra de las funcionalidades de las acciones de Kirigami es la de proporcionar diversas sugerencias a los elementos que usan acciones sobre cómo deben mostrar la acción. Esto se controla principalmente mediante las propiedades ` displayHint y displayComponent .
Estas propiedades serán respetadas por el elemento siempre que sea posible. Por ejemplo, la siguiente acción se mostrará como un TextField con el elemento haciendo todo lo posible para mantenerse visible el mayor tiempo posible.
import org.kde.kirigami as Kirigami
Kirigami.Action {
text: "Search"
icon.name: "search"
displayComponent: TextField { }
displayHint: Kirigami.DisplayHints.KeepVisible
}
Uso de acciones en otros componentes
As mentioned in the introduction tutorial for actions, Kirigami Actions are contextual, which means they show up in different places depending on where you put them. In addition to that, they also have different representations for desktop and mobile.
Página
A Kirigami.Page shows Actions on the right of the top header in desktop mode, and on a footer in mobile mode.
|
|
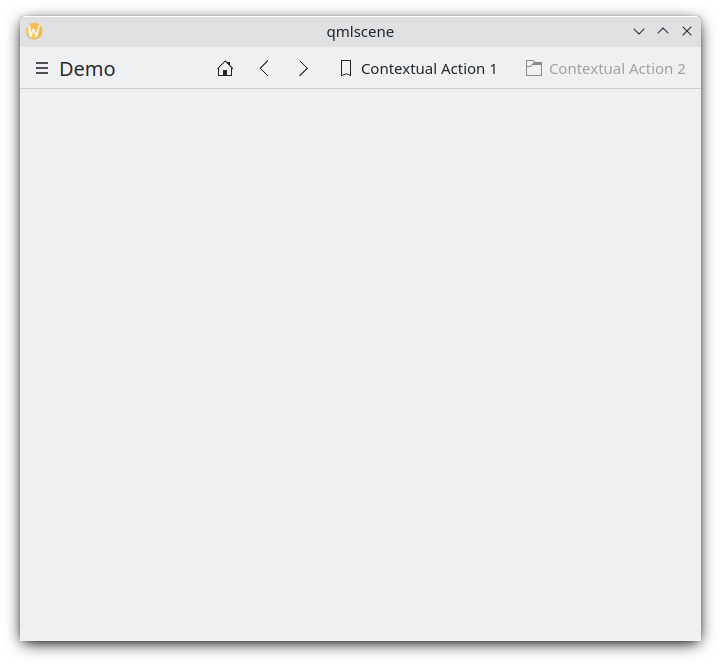
Acciones de página en el escritorio
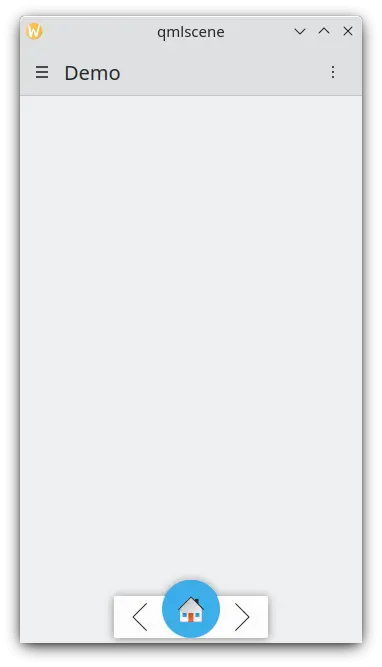
Acciones de página en un dispositivo móvil
Cajón global
The Kirigami.GlobalDrawer is a menu-like sidebar that provides an action based navigation to your application. This is where nested actions are useful because it is possible to create nested navigation:
import org.kde.kirigami as Kirigami
Kirigami.ApplicationWindow {
title: "Actions Demo"
globalDrawer: Kirigami.GlobalDrawer {
title: "Demo"
titleIcon: "applications-graphics"
actions: [
Kirigami.Action {
text: "View"
icon.name: "view-list-icons"
Kirigami.Action {
text: "View Action 1"
onTriggered: showPassiveNotification("View Action 1 clicked")
}
Kirigami.Action {
text: "View Action 2"
onTriggered: showPassiveNotification("View Action 2 clicked")
}
},
Kirigami.Action {
text: "Action 1"
onTriggered: showPassiveNotification("Action 1 clicked")
},
Kirigami.Action {
text: "Action 2"
onTriggered: showPassiveNotification("Action 2 clicked")
}
]
}
//...
}
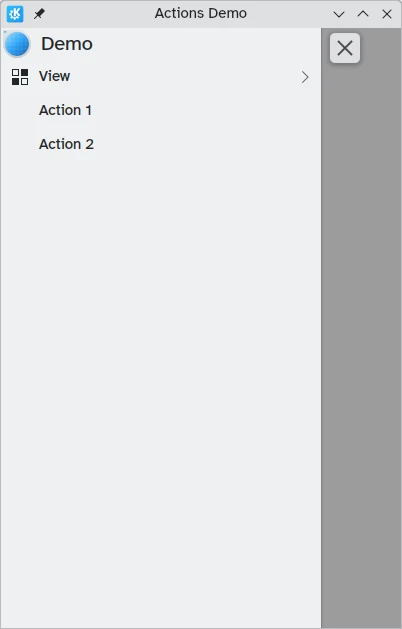
Acciones del cajón global en el escritorio
Puede obtener más información sobre los cajones globales en la página de documentación de los cajones.
Cajón de contexto
A Kirigami.ContextDrawer consists of an additional set of actions that are hidden behind a three-dots menu on the top right in desktop mode or on the bottom right in mobile mode if there is no space. It is used to display actions that are only relevant to a specific page. You can read more about them in our Kirigami Drawers tutorial.
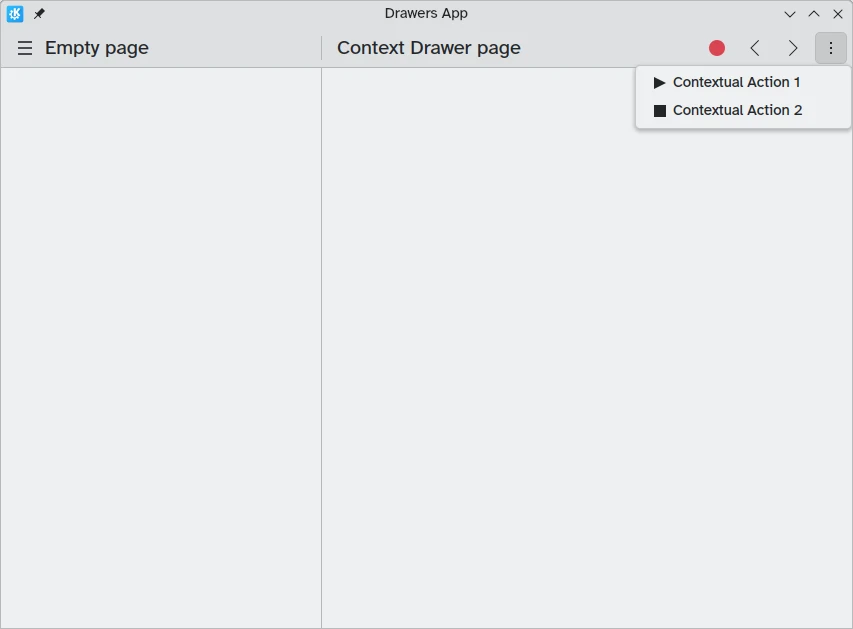

ActionTextFields
Una Kirigami.ActionTextField se usa para añadir algunas acciones de contexto a un campo de teto. Por ejemplo, para borrar el texto o para buscar dicho texto.
Kirigami.ActionTextField {
id: searchField
rightActions: [
Kirigami.Action {
icon.name: "edit-clear"
visible: searchField.text !== ""
onTriggered: {
searchField.text = ""
searchField.accepted()
}
}
]
}
En este ejemplo, vamos a crear un botón de borrado para un campo de búsqueda, que solo será visible tras introducir texto.
Nota
Raramente debería usar un ActionTextField directamente. Tanto SearchField como PasswordField heredan deActionTextField
y es probable que cubran sus necesidades.SwipeListItem
Un Kirigami.SwipeListItem es un delegado diseñado para permitir acciones adicionales. Cuando se usa un ratón, siempre se mostrarán sus acciones. En un dispositivo táctil, se mostrará al arrastrar el elemento mediante un asa. En las siguientes imágenes, son los iconos de la derecha.
ListView {
model: myModel
delegate: SwipeListItem {
Controls.Label {
text: model.text
}
actions: [
Action {
icon.name: "document-decrypt"
onTriggered: print("Action 1 clicked")
},
Action {
icon.name: model.action2Icon
onTriggered: //hacer algo
}
]
}
}
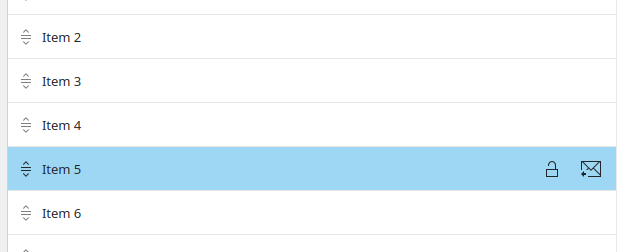
SwipeListItem en una computadora
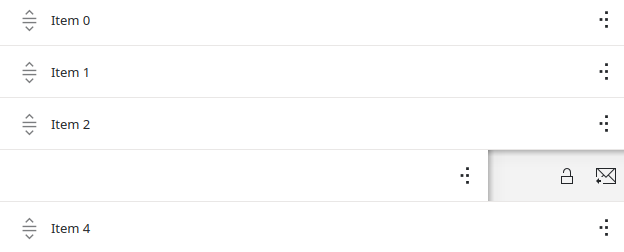
SwipeListItem en un dispositivo móvil
ActionToolBar
Una Kirigami.ActionToolBar es una barra de herramientas creada a partir de una lista de acciones. De forma predeterminada, cada acción que quepa en la barra de herramientas se representará mediante un ToolButton , mientras que las que no quepan se moverán a un menú en un extremo de la barra de herramientas.
Como ActionTextField , no necesita usar ActionToolBar directamente, ya que la usan las cabeceras de las páginas y de las tarjetas para mostrar sus acciones.
import org.kde.kirigami as Kirigami
Kirigami.ApplicationWindow {
title: "Actions Demo"
width: 350
height: 350
header: Kirigami.ActionToolBar {
actions: [
Kirigami.Action {
text: i18n("View Action 1")
onTriggered: showPassiveNotification(i18n("View Action 1 clicked"))
},
Kirigami.Action {
text: i18n("View Action 2")
onTriggered: showPassiveNotification(i18n("View Action 2 clicked"))
},
Kirigami.Action {
text: i18n("Action 1")
onTriggered: showPassiveNotification(i18n("Action 1 clicked"))
},
Kirigami.Action {
text: i18n("Action 2")
onTriggered: showPassiveNotification(i18n("Action 2 clicked"))
}
]
}
}

Una barra de herramientas horizontal que se muestra en la parte superior de la aplicación
Puede leer más sobre los componentes ActionToolBar en su propia página de documentación.
Tarjetas
Una
Kirigami.Card
se usa para mostrar una colección de información o acciones juntas. Dichas acciones se pueden añadir al grupo actions
, del mismo modo que los componentes anteriores.
Kirigami.Card {
actions: [
Kirigami.Action {
text: qsTr("Action1")
icon.name: "add-placemark"
},
Kirigami.Action {
text: qsTr("Action2")
icon.name: "address-book-new-symbolic"
},
// ...
]
banner {
source: "../banner.jpg"
title: "Title Alignment"
titleAlignment: Qt.AlignLeft | Qt.AlignBottom
}
contentItem: Controls.Label {
wrapMode: Text.WordWrap
text: "My Text"
}
}
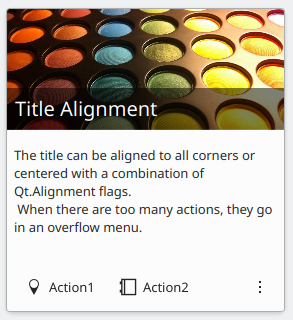
Para más información, consulte la página del componente de tarjetas.