Koloroj kaj etosoj en Kirigami
Kirigami havas kolorpaletron, kiu sekvas la sistemajn kolorojn por pli bone integriĝi kun la platformo, sur kiu ĝi funkcias (t.e. Plasma Desktop, Plasma Mobile, GNOME, Android, ktp.).
Ĉiuj QML-komponentoj de Kirigami kaj QtQuick Controls jam devus sekvi ĉi tiun paletron defaŭlte, do kutime neniu propra kolorigo devus esti bezonata por ĉi tiuj regiloj.
Primivaj komponantoj kiel Rektangulo ĉiam estu kolorigitaj per la kolorpaletro provizita de Kirigami per la kuna propreco Kirigami.Theme.
Malmolkodigitaj koloroj en QML, kiel ekzemple #32b2fa
aŭ ruĝa
, devus kutime esti evititaj; se vere necesas havi elementojn kun kutimaj koloroj, ĝi devus esti areo kie nur kutimaj koloroj estas uzataj (kutime en la enhavareo de la apo, kaj neniam en kromaj areoj kiel ilobretoj aŭ dialogoj). Ekzemple, malmola kodita nigra
malfono ne povas esti uzata super fono Kirigami.Theme.backgroundColor, ĉar se la platformo uzas malhelan kolorskemon la rezulto havos malbonan kontraston kun nigra super preskaŭ nigra. Ĉi tio estas problemo de alirebleco kaj devus esti evitita.
Noto
Se vi vere bezonas uzi proprajn kolorojn, kontrolu Kontrast por certigi, ke la koloroj, kiujn vi elektas, havas bonan kontraston kaj konformas al WCAG. .wikipedia.org/wiki/Web_Content_Accessibility_Guidelines).Etoso
Kirigami.Theme estas kuna propreco, kaj tial ĝi estas uzebla por iu ajn QML-aĵo. Ĝiaj proprecoj inkluzivas ĉiujn kolorojn disponeblajn en la paletro, kaj kian paletron uzi, kiel ekzemple la propreco colorSet.
import QtQuick
import org.kde.kirigami as Kirigami
Kirigami.ApplicationWindow {
height: 300
width: 400
pageStack.initialPage: Kirigami.Page {
Rectangle {
anchors.centerIn: parent
implicitHeight: 100
implicitWidth: 200
color: Kirigami.Theme.highlightColor
}
}
}
Kirigami Gallery disponigas kodekzemplon montrantan [ĉiujn kolorojn disponeblajn por Kirigami](https://invent.kde.org/sdk/kirigami-gallery/- /blob/master/src/data/contents/ui/gallery/ColorsGallery.qml) tra Kirigami.Theme. Ĉi tio inkluzivas ĉiujn iliajn statojn: se vi alklakas ekster la fenestro, la koloroj ŝanĝas al sia neaktiva stato, kaj se vi ŝanĝas vian sistemon al malhela temo, la malhelaj variantoj de la koloroj aperu en reala tempo.
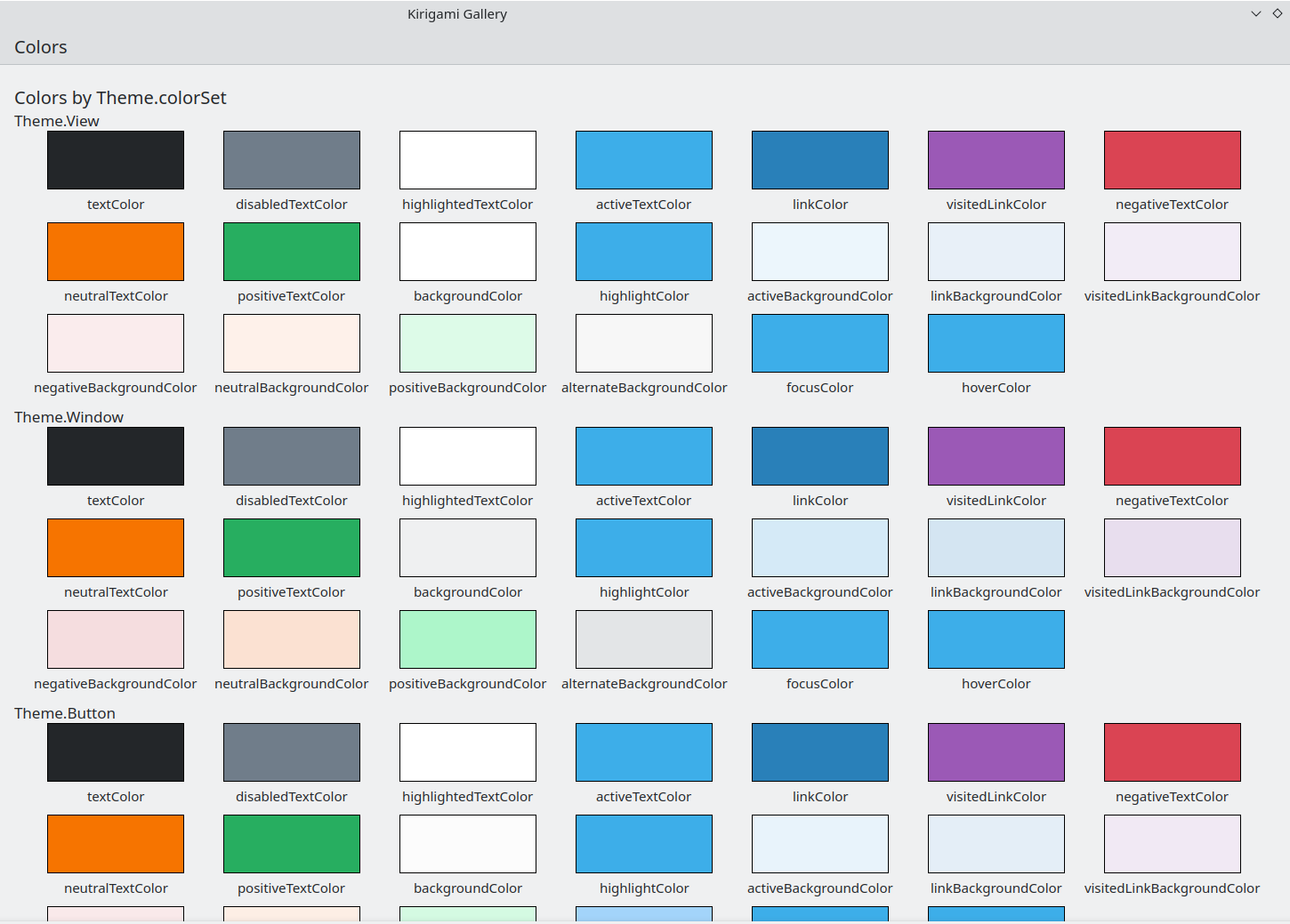
La Koloroj-komponento en Kirigami Gallery
Koloro Aro
Depende de kie troviĝas regilo, ĝi devus uzi malsaman koloraron: ekzemple, kiam la kolorskemo de Breeze Light estas uzata en [Vidoj](https://doc.qt.io/qt-6/qtquick-modelviewsdata- modelview.html), la normala fono estas preskaŭ blanka, dum en aliaj regionoj, kiel ilobretoj aŭ dialogoj, la normala fonkoloro estas griza.
Se vi difinas koloraron por ero, ĉiuj ĝiaj filaj eroj rekursie heredos ĝin aŭtomate (krom se la propreco heredaĵo eksplicite estis agordita al false
, kio ĉiam devas esti farita kiam la programisto volas devigi specifan koloraron) do estas facile ŝanĝi kolorojn por tuta hierarkio de eroj sen tuŝi iun ajn el la eroj mem.
Kirigami.Theme subtenas 5 malsamajn kolorajn arojn:
- Vido: Koloro aro por eroj-vidoj, kutime la plej malpeza el ĉiuj (en helkoloraj etosoj)
- Fenestro: Koloro aro por fenestroj kaj kromaj areoj (ĉi tio ankaŭ estas la defaŭlta koloraro)
- Butono: Koloro uzata de butonoj
- Elekto: Koloro uzata de elektitaj areoj
- Konsileto: Koloro uzata de konsiletoj
- Komplementa: Koloro intencita esti komplementa al Fenestro: kutime malhela eĉ en helaj etosoj. Povas esti uzata por emfazo en malgrandaj areoj de la apliko
Jen ekzemplo montranta kiel koloraroj estas hereditaj kaj povas esti uzataj por distingi malsamajn komponentojn. Granda bordo estis aldonita por kontrasti kolorojn.
|
|
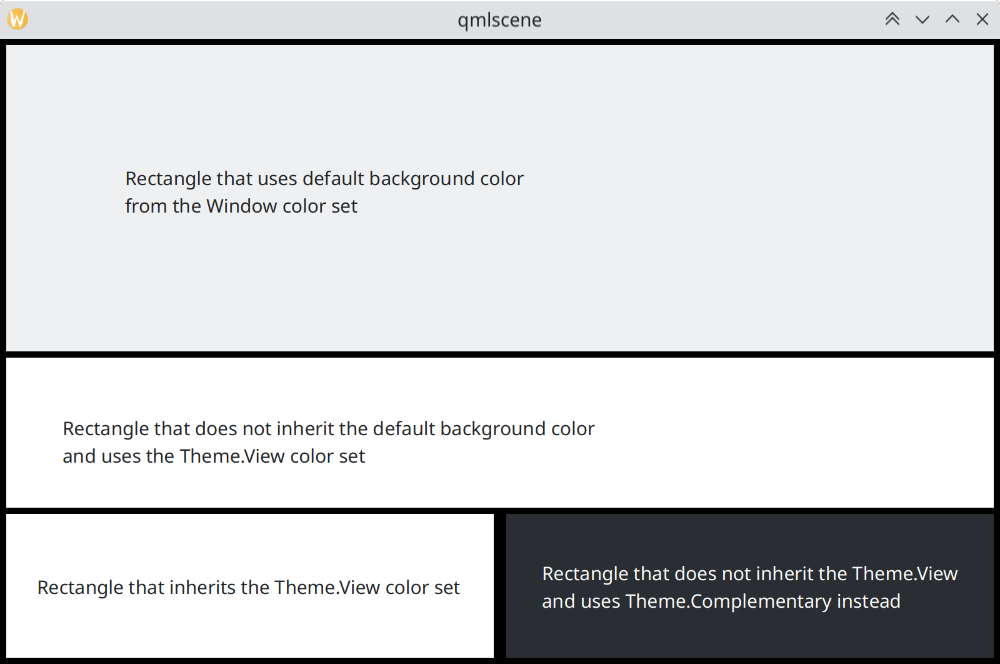
Kiel koloraroj diferencas en Breeze
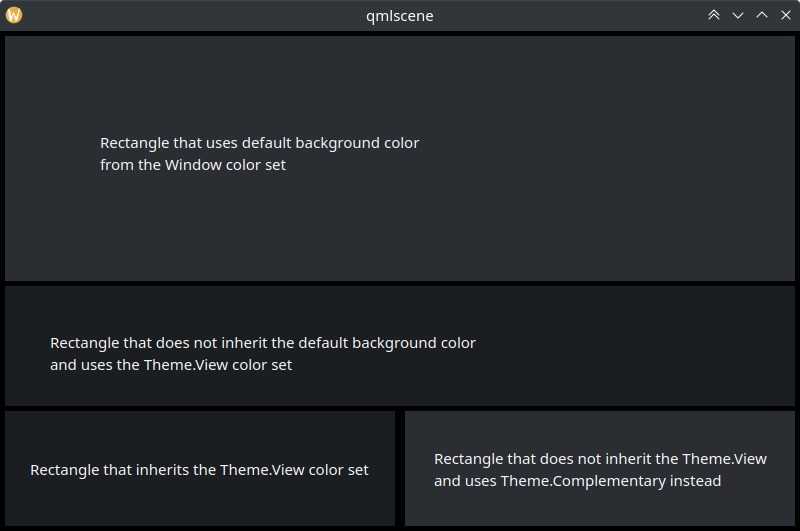
Kiel koloraroj diferencas en Breeze Dark
Uzante Proprajn Kolorojn
Kvankam estas malkuraĝigite uzi malmolkoditajn kolorojn, Kirigami ofertas pli konserveblan manieron asigni propran malmolkoditan paletron al objekto kaj ĉiuj ĝiaj infanoj, kio permesas difini tiajn proprajn kolorojn en unu loko kaj unu nur:
import QtQuick
import org.kde.kirigami as Kirigami
Kirigami.ApplicationWindow {
title: "Custom colors"
height: 300
width: 300
Rectangle {
anchors.fill: parent
Kirigami.Theme.inherit: false
// RIMARKO: sendepende de la koloraro uzita, estas rekomendite antatataŭigi ĉiujn
// disponeblajn kolorojn en Etoso por eviti malbone kontrastantajn kolorojn
Kirigami.Theme.colorSet: Kirigami.Theme.Window
Kirigami.Theme.backgroundColor: "#b9d795"
Kirigami.Theme.textColor: "#465c2b"
Kirigami.Theme.highlightColor: "#89e51c"
// Redifini ĉiujn aliajn kolorojn kiejn vi volas
// Tio ĉi estos "#b9d795"
color: Kirigami.Theme.backgroundColor
Rectangle {
// Tio ĉi estos "#465c2b"
anchors.centerIn: parent
height: Math.round(parent.height / 2)
width: Math.round(parent.width / 2)
color: Kirigami.Theme.textColor
}
}
}
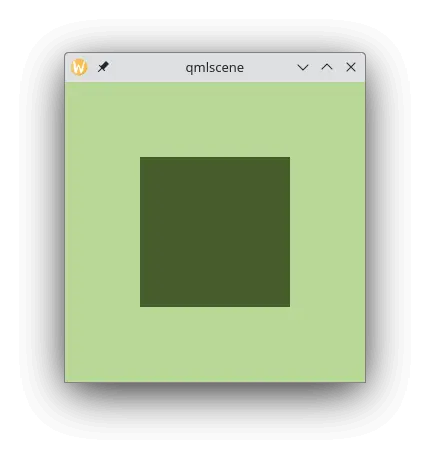
Ekzemplo kun propraj koloroj