Introduction to Kirigami Addons
Kirigami Addons is an additional set of visual components that work well on mobile and desktop and are guaranteed to be cross-platform. It uses Kirigami under the hood to create its components.
Here you will be setting up your new Kirigami Addons project and be introduced to a few useful components.
These components make use of KDE's localization facilities, so before we start using these, we will need to set a little project that makes use of KLocalizedContext .
Setting up your project
The initial project structure will look like so:
addonsexample/
├── CMakeLists.txt
├── main.cpp
├── resources.qrc
└── contents/
└── ui/
└── main.qml
Tip
You can quickly create this file structure with:
mkdir -p addonsexample/contents/ui
touch addonsexample/{CMakeLists.txt,main.cpp,resources.qrc}
touch addonsexample/contents/ui/main.qml
We start by using a very standard CMakeLists.txt
:
cmake_minimum_required(VERSION 3.20)
project(FormCardTutorial)
find_package(ECM REQUIRED NO_MODULE)
set(CMAKE_MODULE_PATH ${ECM_MODULE_PATH})
include(KDEInstallDirs)
include(KDECompilerSettings)
include(KDECMakeSettings)
find_package(Qt${QT_MAJOR_VERSION} REQUIRED COMPONENTS
Widgets # For QApplication
Quick # For QML
QuickControls2 # For QQuickStyle
)
find_package(KF${QT_MAJOR_VERSION} REQUIRED COMPONENTS
CoreAddons # For KAboutData
I18n # For KLocalizedContext
)
add_executable(addonsexample)
target_sources(addonsexample PRIVATE main.cpp resources.qrc)
target_link_libraries(addonsexample PRIVATE
Qt::Widgets
Qt::Quick
Qt::QuickControls2
KF${QT_MAJOR_VERSION}::CoreAddons
KF${QT_MAJOR_VERSION}::I18n
)
install(TARGETS addonsexample DESTINATION ${KDE_INSTALL_BINDIR})
A standard resources.qrc
:
<!DOCTYPE RCC>
<RCC version="1.0">
<qresource prefix="/">
<file alias="main.qml">contents/ui/main.qml</file>
</qresource>
</RCC>
The interesting part will be the main.cpp
:
#include <QtQml>
#include <QApplication>
#include <QQmlApplicationEngine>
#include <QIcon>
#include <QQuickStyle>
#include <KAboutData>
#include <KLocalizedContext>
#include <KLocalizedString>
int main(int argCount, char* argVector[])
{
QApplication app(argCount, argVector);
KLocalizedString::setApplicationDomain("org.kde.addonsexample");
KAboutData aboutData(
QStringLiteral("addonsexample"),
i18nc("@title:window", "Addons Example"),
QStringLiteral("1.0"),
i18nc("@info", "This program shows how to use Kirigami Addons"),
KAboutLicense::GPL_V3,
QStringLiteral("(C) 2023"),
i18nc("@info", "Optional text shown in the About"),
QStringLiteral("https://kde.org"));
aboutData.addAuthor(i18nc("@info:credit", "John Doe"),
i18nc("@info:credit", "Maintainer"));
KAboutData::setApplicationData(aboutData);
if (qEnvironmentVariableIsEmpty("QT_QUICK_CONTROLS_STYLE")) {
QQuickStyle::setStyle(QStringLiteral("org.kde.desktop"));
}
QApplication::setWindowIcon(QIcon::fromTheme(QStringLiteral("kde")));
qmlRegisterSingletonType(
"org.kde.about",
1, 0, "About",
[](QQmlEngine *engine, QJSEngine *) -> QJSValue {
return engine->toScriptValue(KAboutData::applicationData());
}
);
QQmlApplicationEngine engine;
engine.rootContext()->setContextObject(new KLocalizedContext(&engine));
engine.load(QStringLiteral("qrc:/main.qml"));
app.exec();
}
If you have read our KXmlGui tutorial or the last Kirigami tutorial on the Kirigami About page, much of this will seem familiar to you.
We create our application and use KAboutData's default constructor to add the metadata of our application, add ourselves as an author, and then use setApplicationData() to finish the process. For later, we also set an application icon that comes from the system theme.
We then use a lambda in qmlRegisterSingletonType to directly send the metadata of our application to the QML side, exposing its properties.
We then instantiate our QML engine, and set its context to use KDE's KLocalizedContext , used to integrate translated strings, passing the just created engine as a parameter.
We simply load our QML file from the resource file, and now we just need to take care of our initial QML file.
FormCard and FormButtonDelegate
The idea for our app is to design our own Kirigami Addons gallery, showcasing multiple components, one per page. The main page will contain a simple list of buttons in a ColumnLayout, each opening a separate page.
Initially, our contents/ui/main.qml
should look like this:
|
|
We use our handy pageStack to set the initial page to a Kirigami.ScrollablePage .
While we could use a FormLayout together with QtQuick Controls components to achieve our goal, here you will be introduced to FormCard.
The main purpose of a FormCard is to serve as a container for other components while following a color different from the background, in a similar manner to a Kirigami.Card , but for settings windows. You can have multiple FormCards in your application to indicate different sections. Your FormCard is also expected to be a direct child of a ColumnLayout.
Importing org.kde.kirigamiaddons.formcard
makes all FormCard components available to your QML file.
We will have only a single section in our main page, so we add a single FormCard:
|
|
The great thing about FormCard is that it does automatic layouting for you. In other words, just the order of its components is enough to indicate their position inside the FormCard, no Layout attached properties are necessary and you are expected not to use anchors or positioners.
We can simply add a few buttons inside our FormCard:
|
|
That's it! The buttons are not usable just yet, but we are now set up to play with our About pages!
We then build and run it like so:
cmake -B build/ -DCMAKE_INSTALL_PREFIX=~/kde5/usr
cmake --build build/
cmake --install build/
aboutexample
To see other ways to build your application (for example, on Windows), see the Getting Started with Kirigami page.
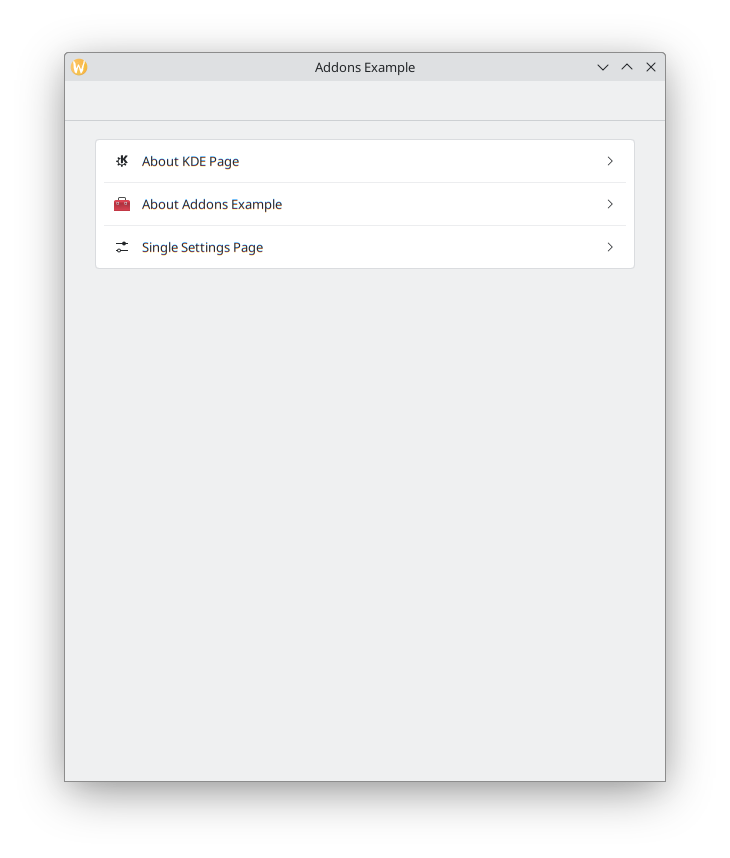