Visual Studio Code
Microsoft Visual Studio Code (VS Code) is a popular cross-platform, general-purpose, open source IDE. Thanks to its powerful extensions ecosystem it supports many languages as well as deep customization options for themes, fonts, keyboard controls, and more.
A screen recording version is available https://www.youtube.com/watch?v=BCJhD57GN0Y
Installation
sudo pacman -S vscode | |
sudo rpm --import https://packages.microsoft.com/keys/microsoft.asc sudo sh -c 'echo -e "[code]\nname=Visual Studio Code\nbaseurl=https://packages.microsoft.com/yumrepos/vscode\nenabled=1\ngpgcheck=1\ngpgkey=https://packages.microsoft.com/keys/microsoft.asc" > /etc/yum.repos.d/vscode.repo' dnf check-update sudo dnf install code |
Setup
The KDE build tool kdesrc-build can automatically generate the configuration files needed for VS Code to work with KDE projects.
To enable this feature, first ensure that kdesrc-build
is installed and
configured; then enable the feature in the kdesrc-build
configuration file
(located at ~/.config/kdesrc-buildrc
by default) - ensure these options are in
the global
section and set to true
:
global
# ... other settings ...
compile-commands-linking true
compile-commands-export true
generate-vscode-project-config true
end global
With these settings, projects built by kdesrc-build
will have the hidden
.vscode
folder created in their source directory; for example, for KCalc this
would be kde/src/kcalc/.vscode
.
The configuration files are generated when a project is built or rebuilt with
kdesrc-build
. If you have already built the project you want to work on
before enabling the generate-vscode-project-config
option, make sure to
rebuild it before opening it in VS Code.
Working on a project
We will use KCalc as an example.
Tip
The Command Palette (Ctrl+Shift+P
) is your friend. It allows you to search for and run commands
and it is a great way to discover features.Opening the project
The project can be opened as a workspace in vs code by opening the src directory as a folder:
File
->Open Folder...
- Select the project's source code directory:
~/kde/src/kcalc
If you have the kdesrc-build
configuration set up as described above, VS Code
will automatically detect the .vscode
folder and load the project with the
correct settings.
The following configuration sections will only need to be done the first time you open a new project in VS Code.
Installing extensions
A notification popup at the bottom-right of the window will ask if you want to install the recommended extensions for working on this project:
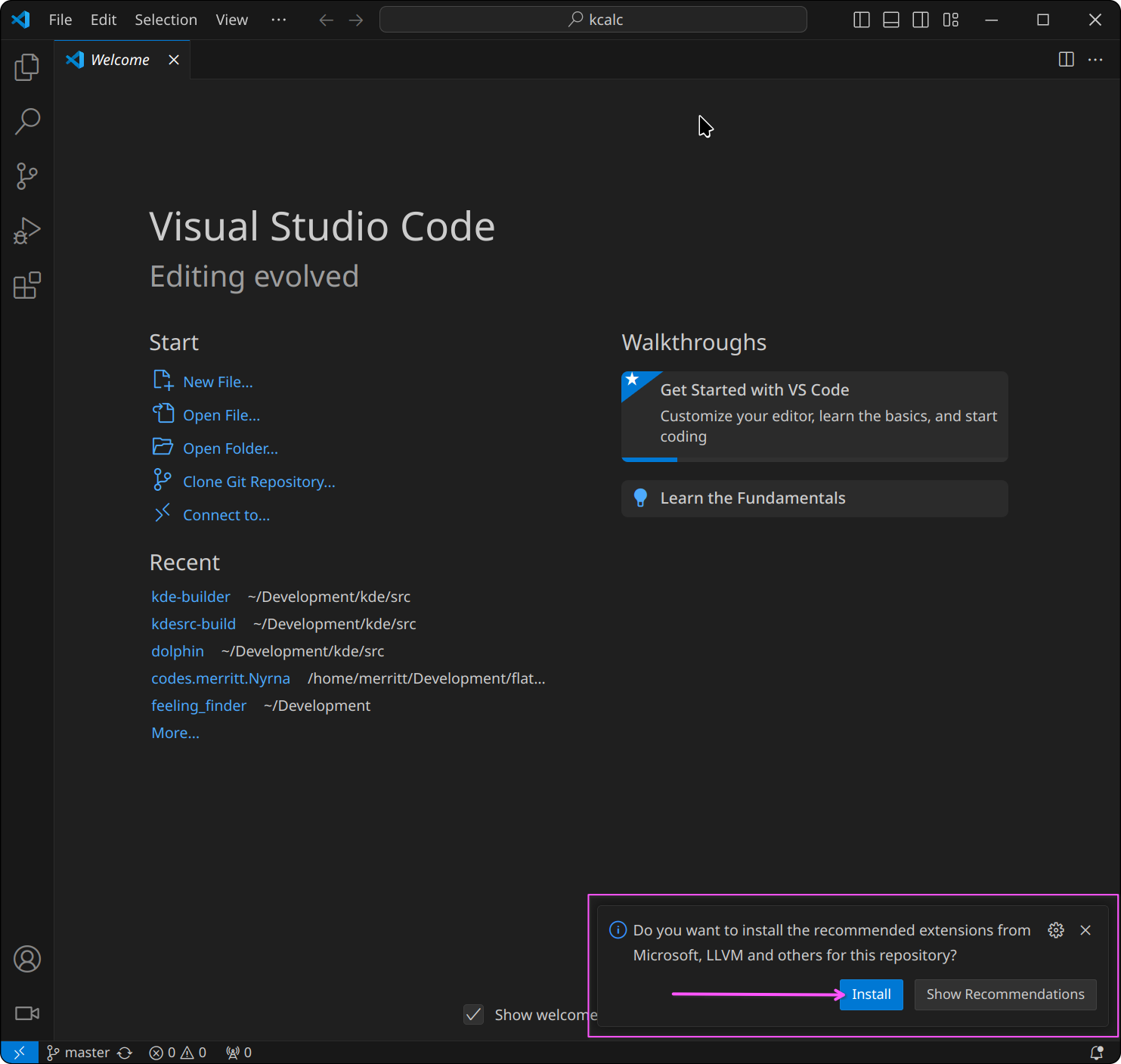
These extensions add support to VS Code for technologies commonly used in KDE projects, such as CMake, C++, Qt, and more.
Click Install
.
Configuring the project
After the extensions have been installed:
- If a notification prompt asks if you want to switch to a pre-release version
of the C++ extension, click
No
. - A notification prompt will ask
Would you like to configure project "kcalc"?
ClickYes
.
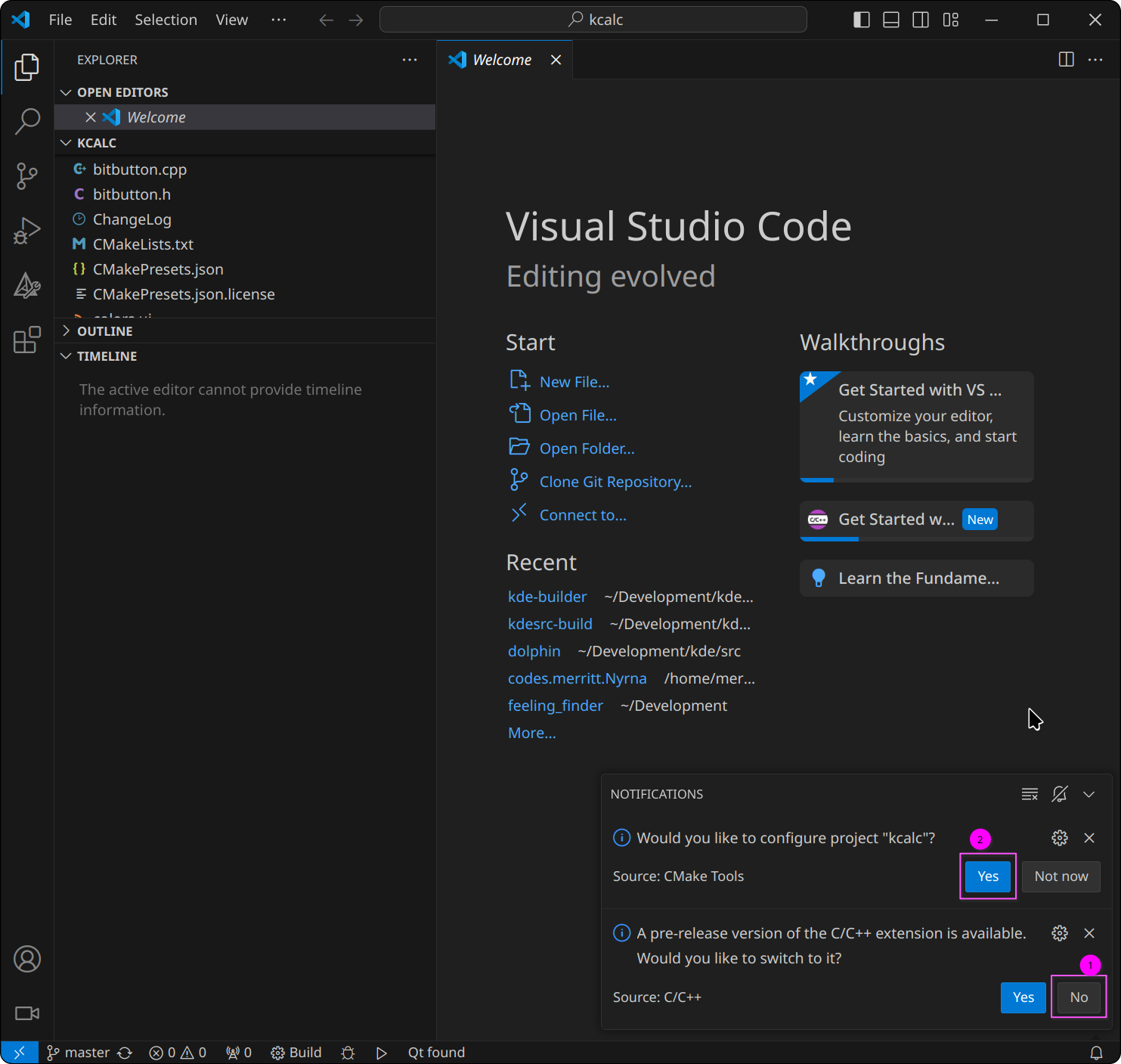
A prompt will open at the top-middle of the window asking to choose a kit
(a set of predefined configurations used when building and running the project). Select
Unspecified
to have the kit chosen automatically based on the project and
system configuration:
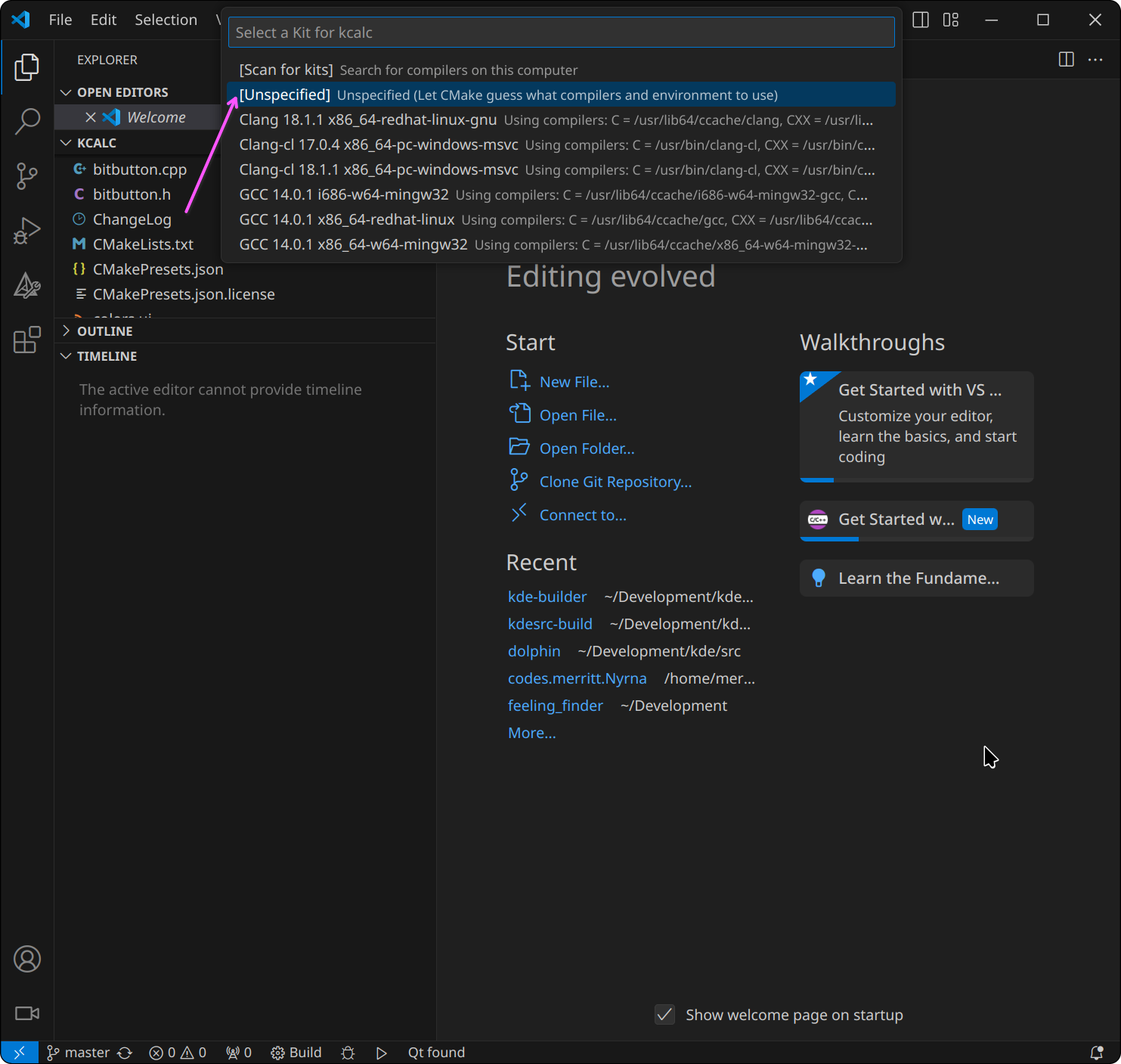
The integrated terminal will open at the bottom of the window, and if the project was configured successfully, the last line should say:
[cmake] -- Build files have been written to: /home/<username>/kde/build/kcalc
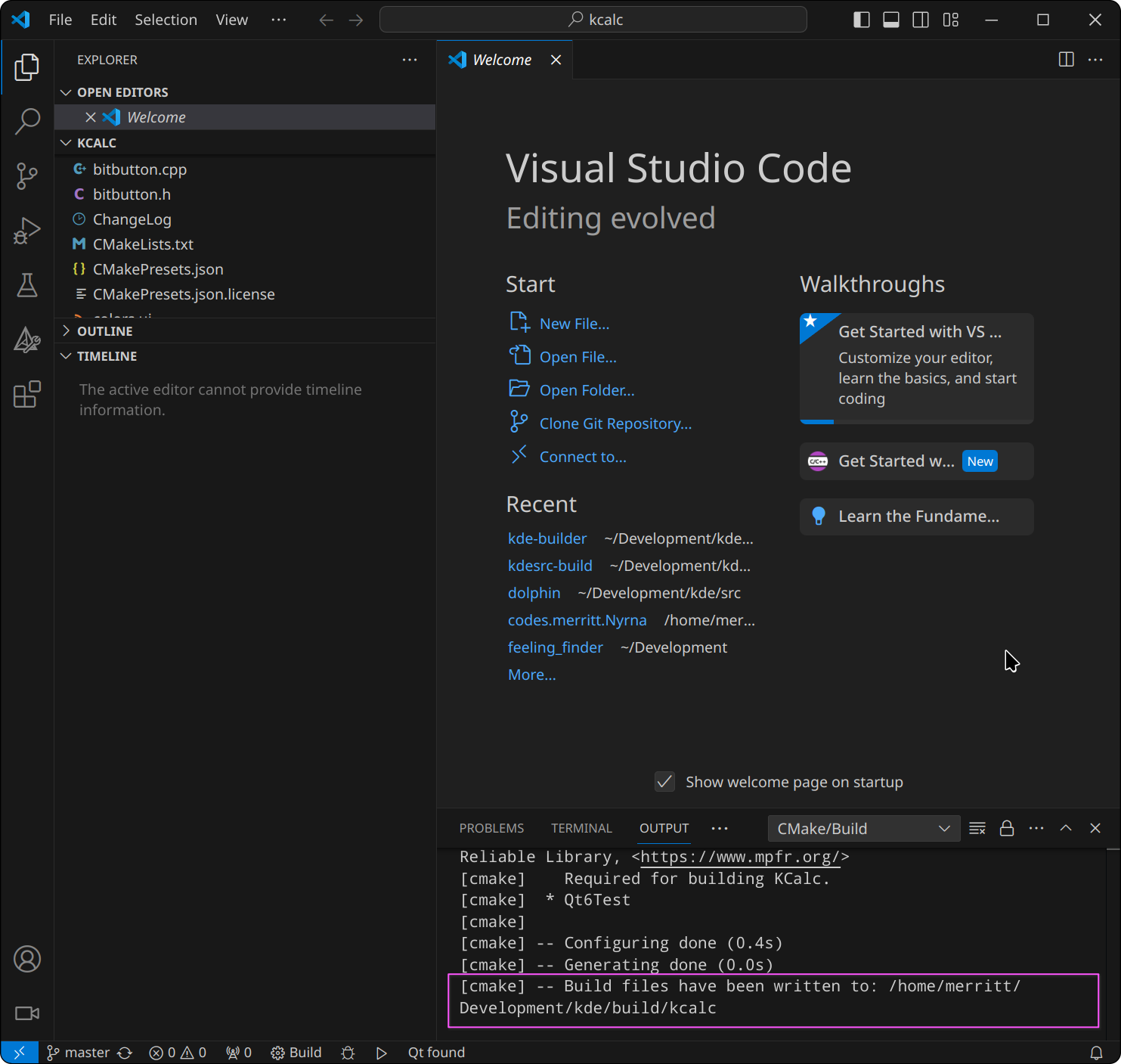
You are ready to start working on the code with VS Code! 🎉
Debugging
To start debugging, click on the Run and Debug
icon on the left sidebar, then
click on the green play button to start debugging.
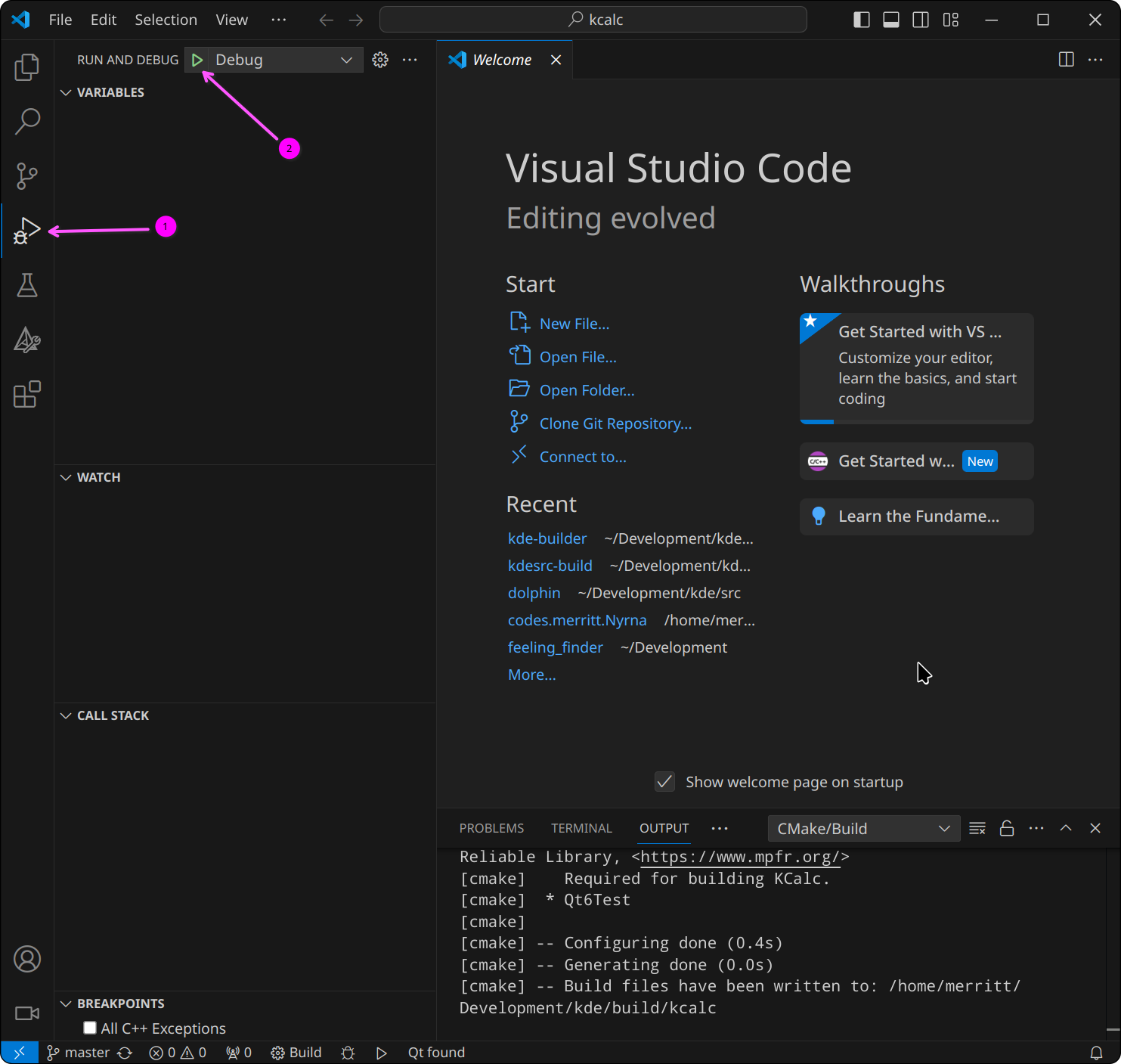
If the project has multiple targets it will open a prompt at the top-middle of
the window asking to choose a target. Select the target you want to debug; in
this case, kcalc
:
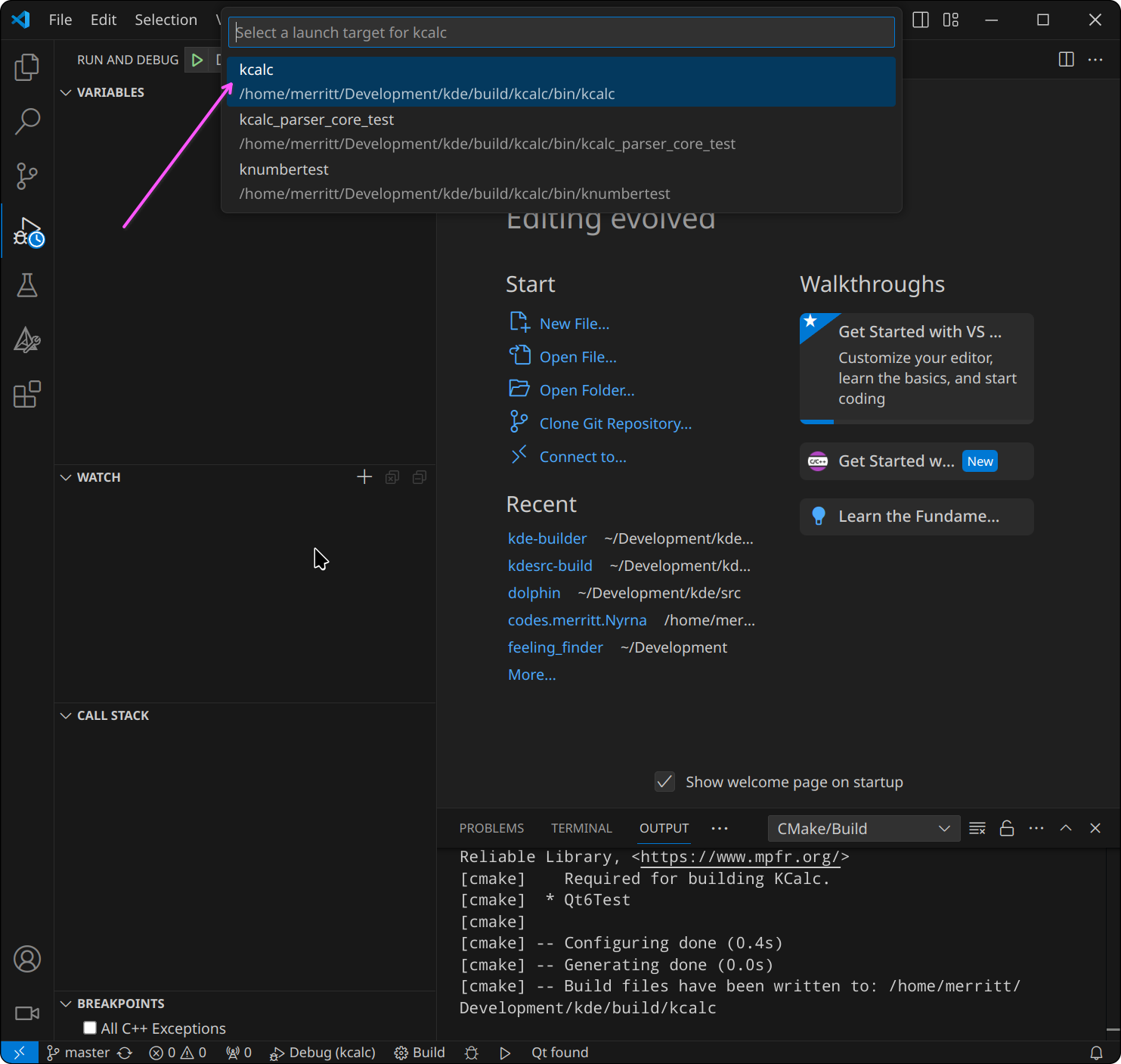
We should now be running a debug session of the kcalc
project. 🚀
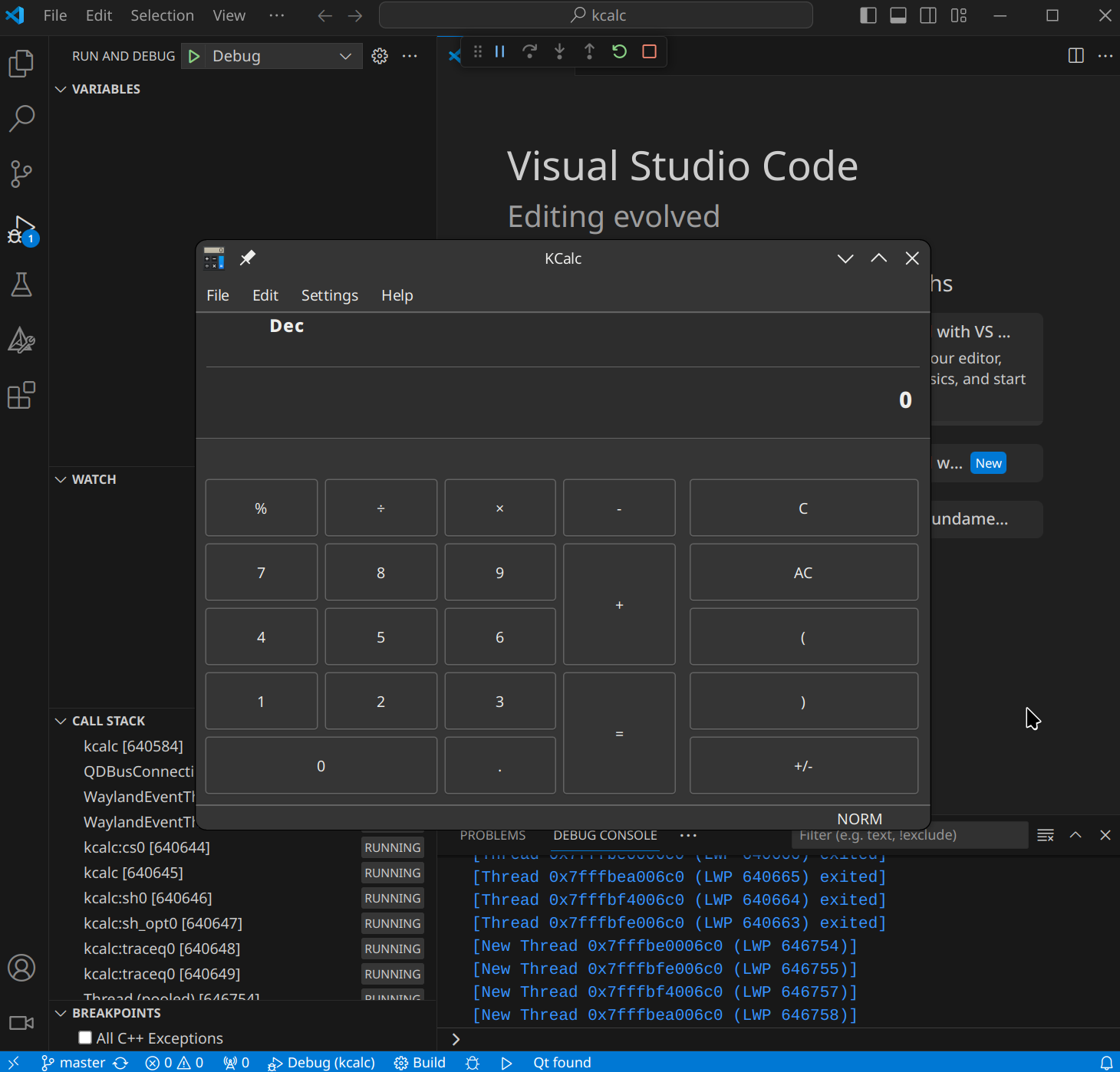
To later change the target, open the Command Palette (Ctrl+Shift+P
) and run
the CMake: Set Debug Target
command.
Debugging KCMs
Note
The configurations for KCMs are only generated by the newerkde-builder
build tool, and not by the legacy kdesrc-build
tool.We'll use the plasma-workspace
project as an example, as it contains many KDE Configuration Modules
(KCMs).
Open the build target selector by clicking the button (defaults to all
):
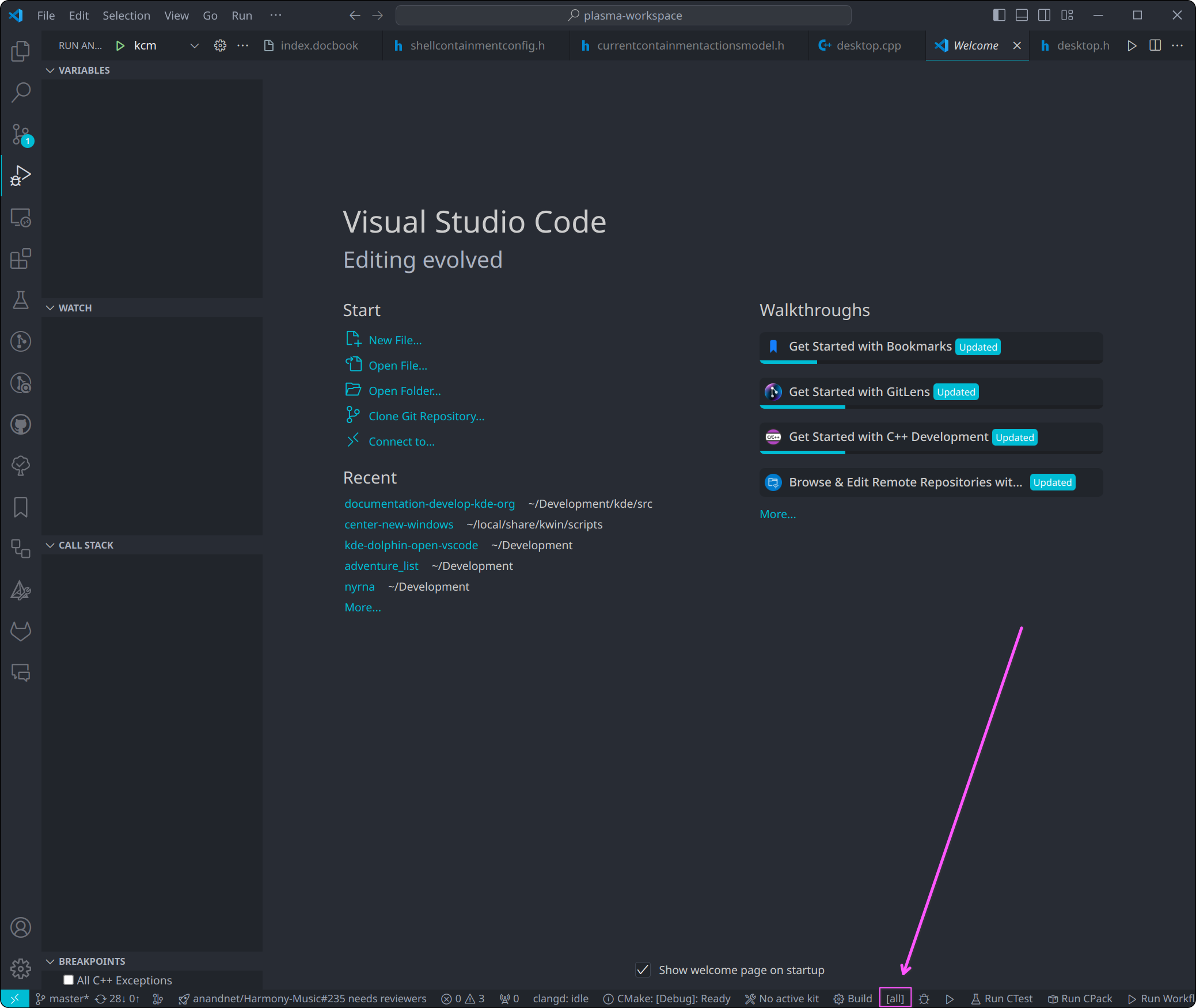
Filter for KCMs by typing kcm_
in the popup, and choose one of the options marked MODULE_LIBRARY
that represents a KCM:
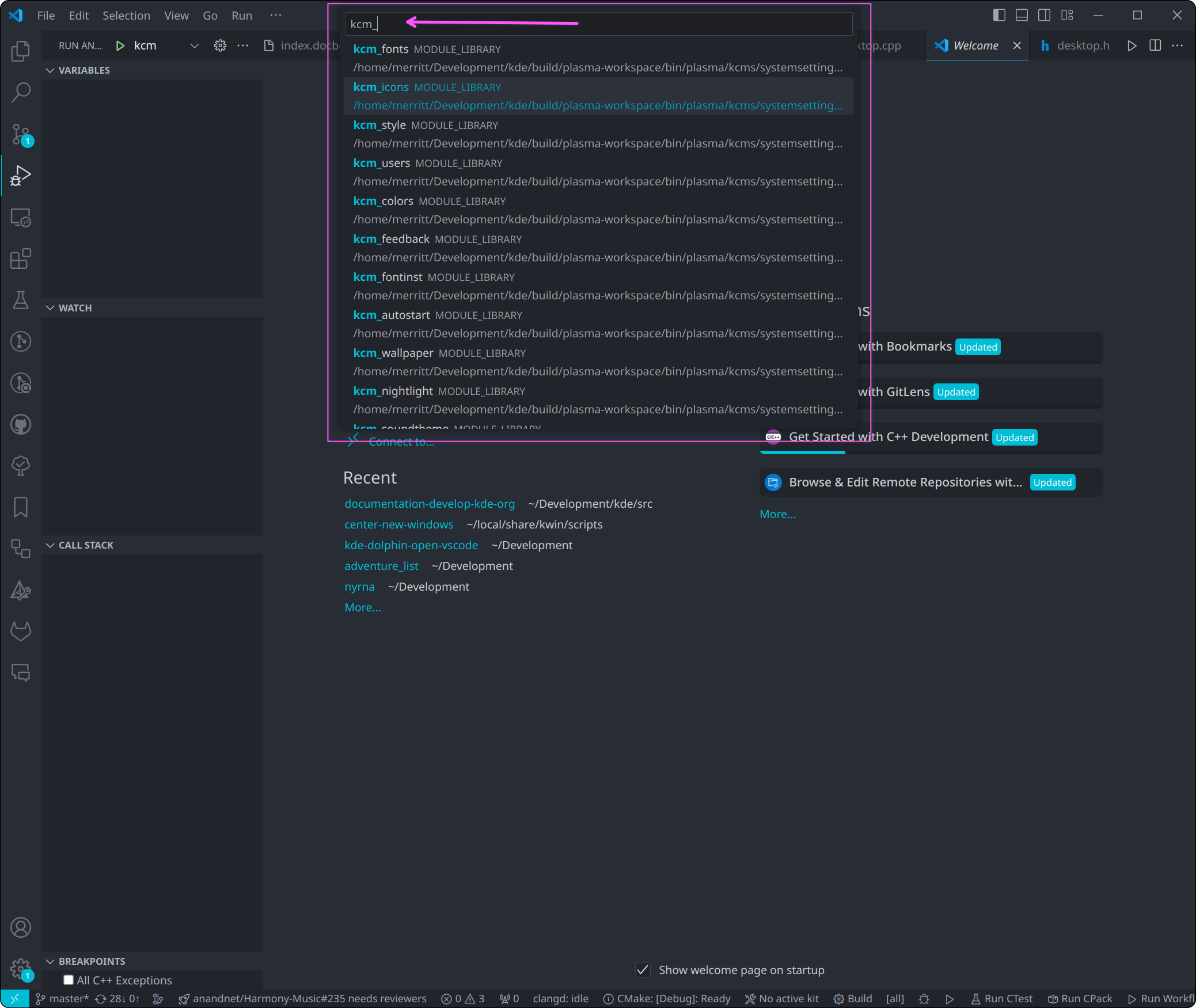
Now you can start debugging the KCM by selecting the kcm
launch configuration and clicking the green play button:
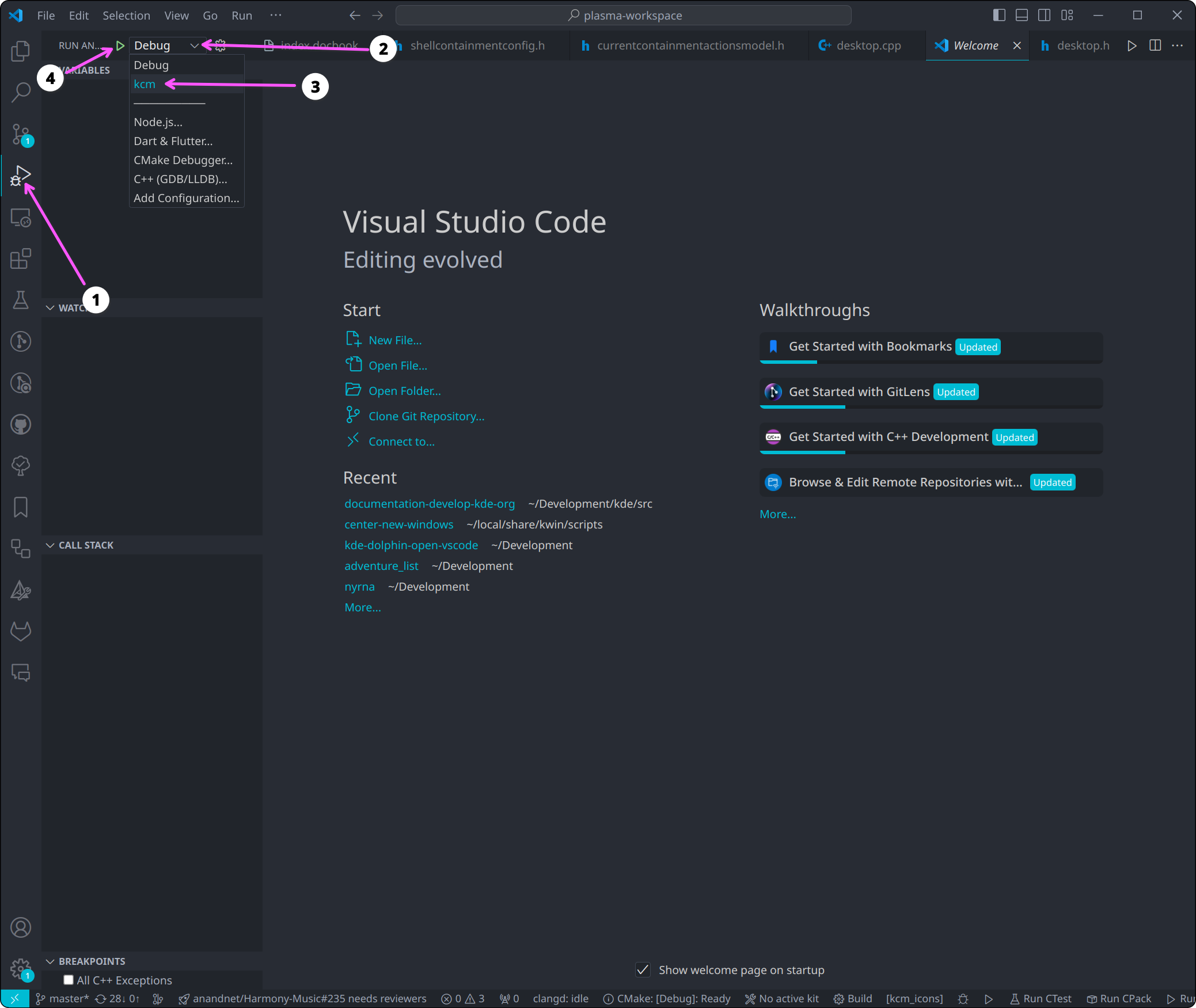
The KCM will open in a new window, and the debugger will hit breakpoints in the C++ code. Breakpoints in QML are sadly not possible.
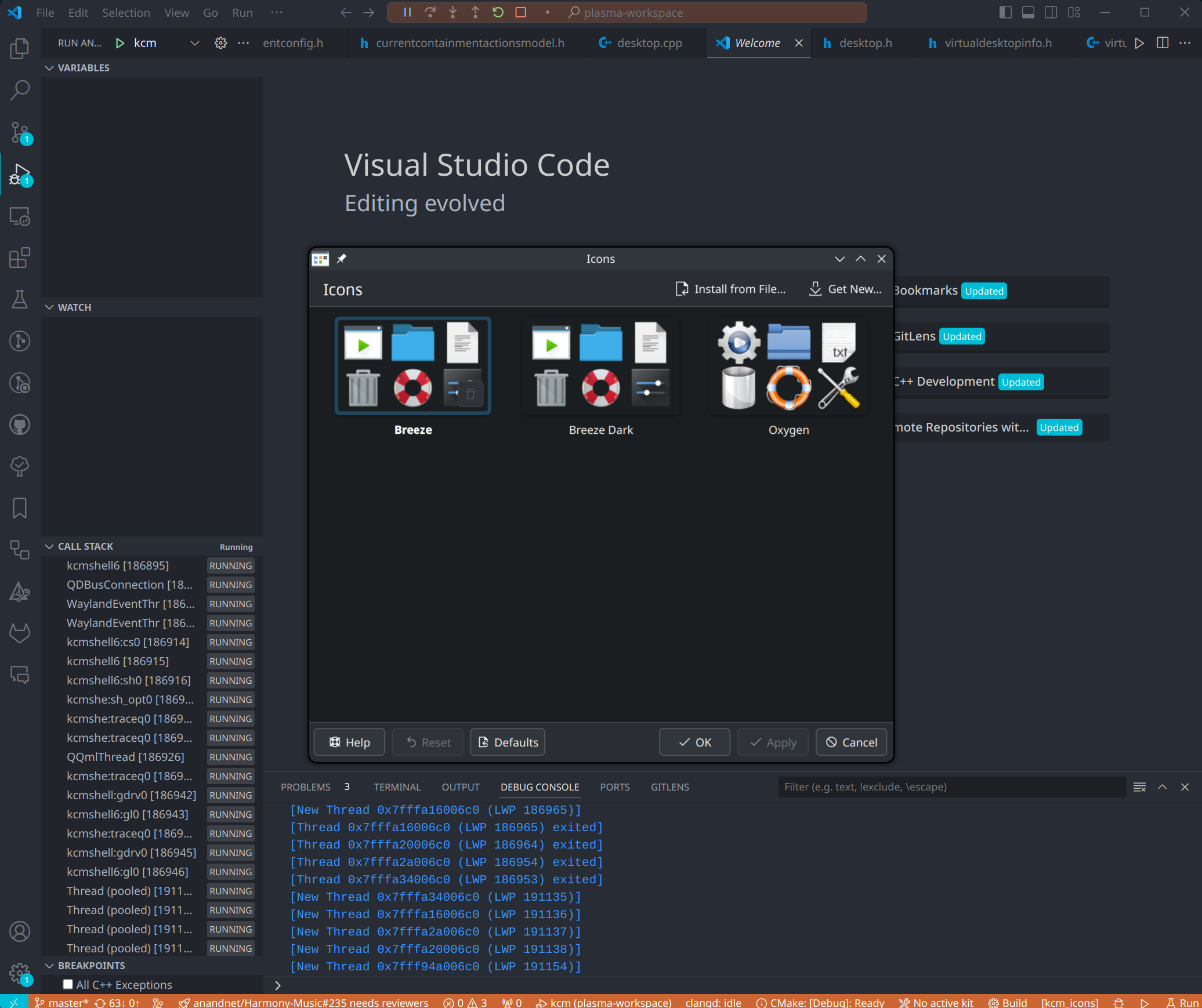
Troubleshooting
- Reloading the window (Command Palette ->
Developer: Reload Window
) can fix some issues, and cause notifications/prompts to reappear if they were missed. - Command Palette ->
CMake: Delete Cache and Reconfigure
orCMake: Reset CMake Tools Extension State (For troubleshooting)
can be useful if things are not working as expected.
Note
In case something goes wrong in config generation, if using kde-builder, you can use the ide_project_configs
logger. Create the file
~/.config/kde-builder-logging.yaml
with the following content:
loggers:
ide_project_configs:
level: DEBUG
handlers: [console-named]
After that, run kde-builder as normal, and you will see corresponding debug messages.
Tips
- The Command Palette
(
Ctrl+Shift+P
) is your friend. It allows you to search for and run commands, and is a great way to discover features. - There is extensive documentation available for VS Code at https://code.visualstudio.com/docs.
- There are first-party video tutorials available at https://code.visualstudio.com/docs/getstarted/introvideos.
Notes
The templates for the .vscode
configuration files are available
here if you
need to reference them or create them manually.